The JavaScript Array indexOf function is a built-in method that returns the index of the first occurrence of a specified element in an array. It takes one required parameter, which is the element to search for, and an optional second parameter, which is the starting index for the search. If the element is found, the function returns the index of the first occurrence of the element. If the element is not found, the function returns -1. This function is useful for quickly finding the position of an element in an array, which can be used for various purposes such as removing or updating the element. Keep reading below to learn how to Javascript Array indexOf in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
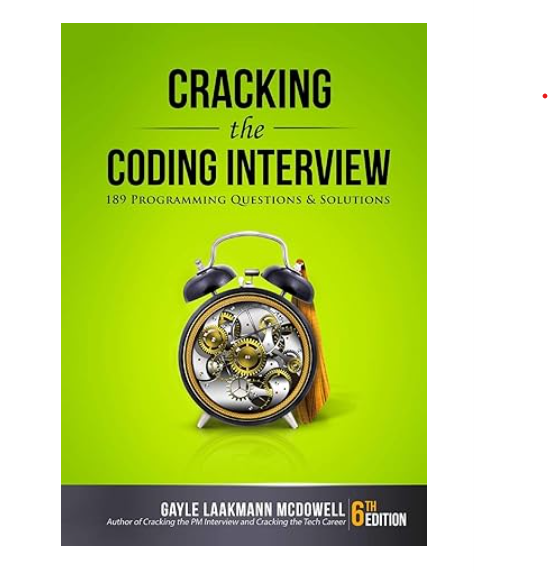
Javascript Array indexOf in C++ With Example Code
JavaScript’s `Array.indexOf()` method is a useful tool for finding the index of a specific element within an array. However, if you’re working with C++, you may be wondering how to achieve the same functionality. Fortunately, there are several ways to implement `indexOf()` in C++.
One approach is to use the `std::find()` function from the `
Here’s an example of how to use `std::find()` to implement `indexOf()`:
#include
#include
template
int indexOf(const std::vector
auto it = std::find(vec.begin(), vec.end(), value);
if (it == vec.end()) {
return -1;
}
return std::distance(vec.begin(), it);
}
In this example, we define a template function `indexOf()` that takes a `std::vector` and a value to search for. We use `std::find()` to search for the value in the vector, and return the index of the value if it’s found. If the value is not found, we return -1.
Another approach is to use a loop to iterate over the elements of the array and compare each element to the value we’re searching for. Here’s an example of how to implement `indexOf()` using a loop:
template
int indexOf(const T* arr, size_t size, const T& value) {
for (size_t i = 0; i < size; i++) {
if (arr[i] == value) {
return i;
}
}
return -1;
}
In this example, we define a template function `indexOf()` that takes a pointer to an array, the size of the array, and a value to search for. We use a loop to iterate over the elements of the array, and return the index of the value if it's found. If the value is not found, we return -1.
Both of these approaches provide a way to implement `indexOf()` in C++. Choose the one that best fits your needs and coding style.
Equivalent of Javascript Array indexOf in C++
In conclusion, the equivalent function of Javascript's Array indexOf in C++ is the std::find function. Both functions serve the same purpose of searching for a specific element in an array and returning its index. However, there are some differences in their syntax and usage. While the Array indexOf function is a method of the Array object in Javascript, the std::find function is a part of the C++ Standard Template Library (STL). Additionally, the std::find function returns an iterator to the found element, which can be converted to an index using the std::distance function. Despite these differences, both functions are powerful tools for searching and manipulating arrays in their respective languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |