The JavaScript Array indexOf function is a built-in method that returns the index of the first occurrence of a specified element in an array. It takes one required parameter, which is the element to search for, and an optional second parameter, which is the starting index for the search. If the element is found in the array, the function returns the index of its first occurrence. If the element is not found, the function returns -1. This method is useful for quickly finding the position of an element in an array, which can be used for various purposes such as removing or updating the element. Keep reading below to learn how to Javascript Array indexOf in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
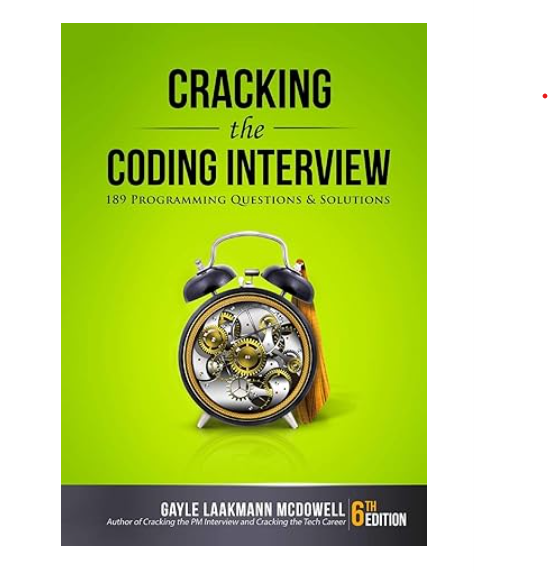
Javascript Array indexOf in Java With Example Code
JavaScript’s `Array.indexOf()` method is a useful tool for finding the index of a particular element within an array. However, if you’re working with Java, you may be wondering how to achieve the same functionality. Fortunately, there is a way to use `Array.indexOf()` in Java.
To use `Array.indexOf()` in Java, you’ll need to first convert your Java array to a JavaScript array. This can be done using the `JSObject.getWindow()` method, which returns a reference to the JavaScript window object. From there, you can use the `eval()` method to execute JavaScript code that converts your Java array to a JavaScript array.
Once you have your JavaScript array, you can use the `indexOf()` method just as you would in JavaScript. Here’s an example:
import netscape.javascript.JSObject;
public class Example {
public static void main(String[] args) {
// Create a Java array
String[] arr = {"apple", "banana", "orange"};
// Get a reference to the JavaScript window object
JSObject window = JSObject.getWindow(null);
// Convert the Java array to a JavaScript array
Object jsArr = window.eval("Array.from(" + Arrays.toString(arr) + ")");
// Use indexOf() on the JavaScript array
int index = (int) window.call("Array.prototype.indexOf", jsArr, "banana");
System.out.println(index); // Output: 1
}
}
In this example, we create a Java array of strings and then convert it to a JavaScript array using `Array.from()`. We then use `Array.prototype.indexOf()` to find the index of the string “banana” within the array. The result is the index of “banana” within the array, which is 1.
Using `Array.indexOf()` in Java can be a powerful tool for working with arrays. By converting your Java arrays to JavaScript arrays, you can take advantage of the many useful array methods available in JavaScript.
Equivalent of Javascript Array indexOf in Java
In conclusion, the equivalent function of Javascript’s Array indexOf in Java is the indexOf method of the ArrayList class. This method allows us to search for a specific element in an ArrayList and returns the index of the first occurrence of that element. It is important to note that the indexOf method in Java only works with objects and not with primitive data types. Additionally, if the element is not found in the ArrayList, the method returns -1. Overall, the indexOf method in Java is a useful tool for searching and manipulating ArrayLists in Java programming.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |