The JavaScript Array indexOf function is a built-in method that returns the index of the first occurrence of a specified element in an array. It takes one required parameter, which is the element to search for, and an optional second parameter, which is the starting index for the search. If the element is found, the function returns the index of the first occurrence of the element. If the element is not found, the function returns -1. This function is useful for quickly finding the position of an element in an array, which can be used for various purposes such as removing or updating the element. Keep reading below to learn how to Javascript Array indexOf in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
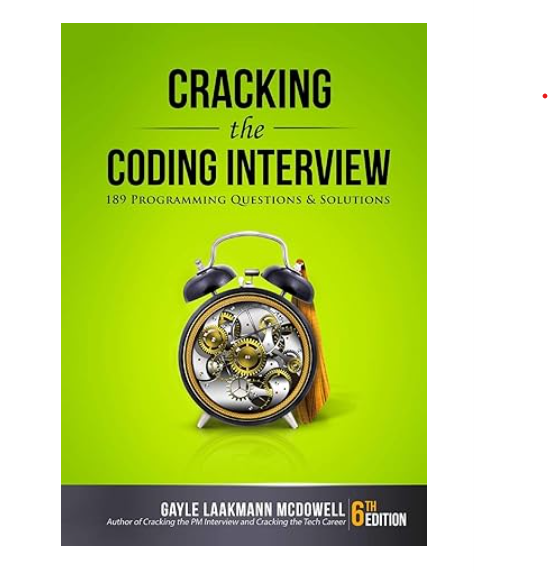
Javascript Array indexOf in Rust With Example Code
JavaScript developers who are transitioning to Rust may find themselves looking for an equivalent to the `indexOf` method for arrays. In JavaScript, `indexOf` returns the first index at which a given element can be found in an array, or -1 if it is not present. Rust provides a similar method for vectors, which are Rust’s equivalent to arrays.
To use the `indexOf` equivalent in Rust, we can call the `iter()` method on the vector to create an iterator, and then use the `position()` method to find the index of the first occurrence of the element we are searching for. If the element is not found, `position()` returns `None`.
Here is an example of how to use `position()` to find the index of an element in a vector:
let vec = vec![1, 2, 3, 4, 5];
let index = vec.iter().position(|&x| x == 3);
match index {
Some(i) => println!("Index of 3: {}", i),
None => println!("3 not found in vector"),
}
In this example, we create a vector of integers and then use `iter()` to create an iterator over the vector. We then call `position()` on the iterator and pass in a closure that checks if the current element is equal to the element we are searching for (in this case, the integer 3). If `position()` finds the element, it returns `Some(index)`, where `index` is the index of the element in the vector. If the element is not found, `position()` returns `None`.
By using the `iter()` and `position()` methods, we can easily find the index of an element in a vector in Rust, which is equivalent to using `indexOf` in JavaScript.
Equivalent of Javascript Array indexOf in Rust
In conclusion, Rust provides a powerful and efficient way to search for elements in an array using the `iter().position()` method. This method is similar to the `indexOf()` function in JavaScript, but with some key differences. Rust’s `iter().position()` method returns an `Option` type, which allows for more robust error handling and avoids the potential pitfalls of returning -1 as in JavaScript. Additionally, Rust’s method is more performant due to its use of iterators and the fact that it does not need to iterate over the entire array to find the desired element. Overall, Rust’s `iter().position()` method is a great alternative to the `indexOf()` function in JavaScript and is a valuable tool for any Rust developer working with arrays.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |