The JavaScript Array join() function is used to join all the elements of an array into a string. It takes an optional separator parameter that specifies the character(s) to be used to separate the elements in the resulting string. If no separator is specified, a comma is used by default. The join() function does not modify the original array, but returns a new string that contains all the elements of the array joined together. This function is commonly used to convert an array into a string that can be easily displayed or transmitted over a network. Keep reading below to learn how to Javascript Array join in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
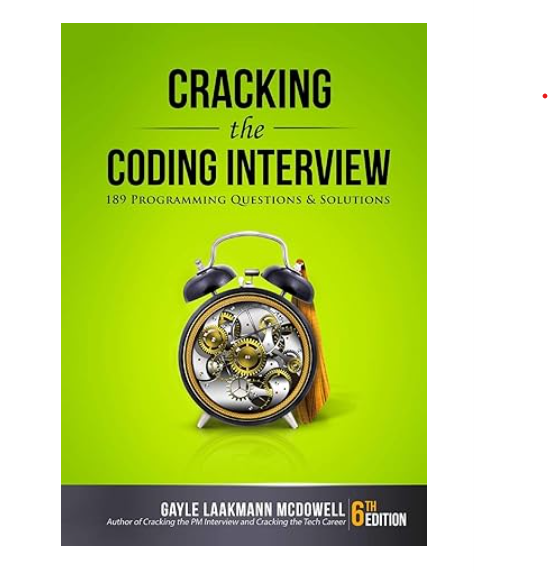
Javascript Array join in Go With Example Code
JavaScript’s `Array.join()` method is a useful tool for combining the elements of an array into a single string. But what if you’re working in Go and need to achieve the same result? Fortunately, Go provides a similar function that can accomplish this task.
The `strings.Join()` function in Go takes two arguments: the first is the slice of strings you want to join, and the second is the separator you want to use between each element. Here’s an example:
package main
import (
"fmt"
"strings"
)
func main() {
mySlice := []string{"apple", "banana", "cherry"}
result := strings.Join(mySlice, ", ")
fmt.Println(result)
}
In this example, we create a slice of strings called `mySlice` containing three elements. We then use `strings.Join()` to join these elements together with a comma and space separator. The resulting string is then printed to the console.
It’s worth noting that `strings.Join()` is not limited to just joining slices of strings. It can also be used to join any type of slice, as long as you convert the elements to strings first. For example:
package main
import (
"fmt"
"strings"
)
func main() {
mySlice := []int{1, 2, 3}
stringSlice := make([]string, len(mySlice))
for i, v := range mySlice {
stringSlice[i] = fmt.Sprint(v)
}
result := strings.Join(stringSlice, ", ")
fmt.Println(result)
}
In this example, we create a slice of integers called `mySlice`. We then create a new slice of strings called `stringSlice` with the same length as `mySlice`. We use a `for` loop to iterate over `mySlice` and convert each element to a string using `fmt.Sprint()`. We then use `strings.Join()` to join the resulting string slice with a comma and space separator.
Overall, `strings.Join()` is a powerful function in Go that can help you easily join slices of any type into a single string.
Equivalent of Javascript Array join in Go
In conclusion, the equivalent function of Javascript’s Array join() in Go is the strings.Join() function. This function takes in a slice of strings and a separator string as arguments and returns a single string that is the concatenation of all the elements in the slice, separated by the separator string. Using the strings.Join() function in Go can make it easier to manipulate and format strings in your code. It is a simple and efficient way to join together elements in a slice and create a single string output. Overall, understanding the equivalent functions in different programming languages can help developers to write more efficient and effective code. By knowing the equivalent of Javascript’s Array join() in Go, developers can easily transition between the two languages and create more robust applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |