The JavaScript Array join() function is used to join all the elements of an array into a string. It takes an optional separator parameter that specifies the character(s) to be used to separate the elements in the resulting string. If no separator is specified, a comma is used by default. The join() function does not modify the original array, but returns a new string that contains all the elements of the array joined together. This function is commonly used to convert an array into a string that can be easily displayed or transmitted over a network. Keep reading below to learn how to Javascript Array join in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
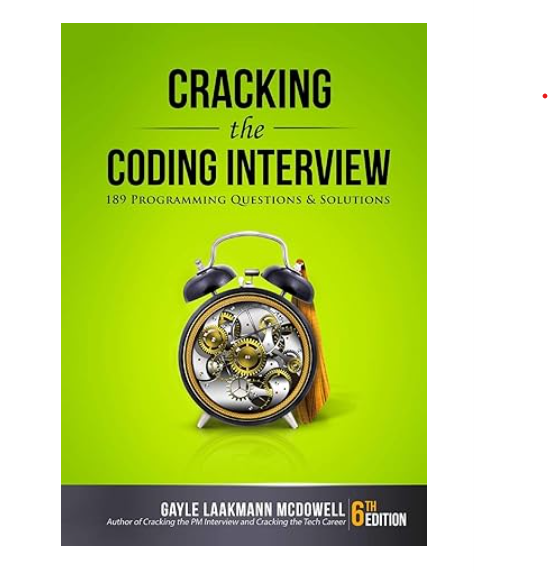
Javascript Array join in Python With Example Code
JavaScript and Python are two popular programming languages used for web development. While they have many similarities, there are also some differences in syntax and functionality. One area where this is evident is in the way arrays are joined. In JavaScript, the `join()` method is used to join all elements of an array into a string. In Python, the `join()` method is used to join elements of a list into a string. However, with a little bit of creativity, you can achieve the same result in Python as you would in JavaScript.
To join elements of a list in Python, you can use the `join()` method of a string. This method takes an iterable (such as a list) and joins all elements into a single string, separated by the string on which the method is called. For example:
my_list = ['apple', 'banana', 'cherry']
separator = ', '
result = separator.join(my_list)
print(result)
This code will output: `apple, banana, cherry`
In this example, we create a list of fruits and a separator string. We then call the `join()` method on the separator string, passing in the list of fruits as an argument. The result is a string with all elements of the list joined together, separated by the separator string.
It’s important to note that the `join()` method can only be called on a string, not on a list. Therefore, we need to create a string object to call the method on. We can do this by using an empty string as the separator:
my_list = ['apple', 'banana', 'cherry']
result = ''.join(my_list)
print(result)
This code will output: `applebananacherry`
In this example, we call the `join()` method on an empty string, passing in the list of fruits as an argument. The result is a string with all elements of the list joined together, with no separator.
In conclusion, while the syntax for joining arrays in JavaScript and lists in Python may be different, the end result can be achieved in both languages. By using the `join()` method of a string in Python, you can easily join elements of a list into a single string, just like you would in JavaScript.
Equivalent of Javascript Array join in Python
In conclusion, the equivalent function to the Javascript Array join() method in Python is the join() method of the string class. Both methods serve the same purpose of joining the elements of an array or list into a single string. However, the syntax and usage of the two methods differ slightly. While the Javascript join() method is called on an array object and takes a separator as an argument, the Python join() method is called on a string object and takes the iterable to be joined as an argument. Despite these differences, both methods are powerful tools for manipulating arrays and strings in their respective languages. As a programmer, it is important to be familiar with the nuances of these methods in order to write efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |