The JavaScript Array join() function is used to join all the elements of an array into a string. It takes an optional separator parameter that specifies the character(s) to be used to separate the elements in the resulting string. If no separator is specified, a comma is used by default. The join() function does not modify the original array, but returns a new string that contains all the elements of the array joined together. This function is commonly used to convert an array into a string that can be easily displayed or transmitted over a network. Keep reading below to learn how to Javascript Array join in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
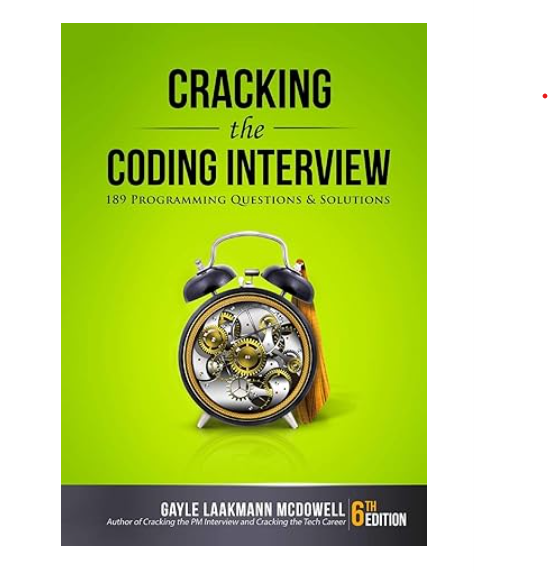
Javascript Array join in Rust With Example Code
JavaScript’s `Array.join()` method is a handy way to concatenate all the elements of an array into a single string, with an optional separator between each element. If you’re working in Rust and need to perform a similar operation on a Rust vector, fear not! Rust’s standard library provides a similar method called `join()`.
To use `join()` on a Rust vector, simply call the method on the vector and pass in the separator as an argument. Here’s an example:
let my_vec = vec!["hello", "world"];
let joined_string = my_vec.join(", ");
println!("{}", joined_string); // prints "hello, world"
In this example, we create a vector called `my_vec` containing two strings. We then call the `join()` method on `my_vec`, passing in `”, “` as the separator. The resulting string is stored in the `joined_string` variable, which we then print to the console.
It’s worth noting that `join()` takes ownership of the vector and returns a new string, rather than modifying the original vector. If you need to join the elements of a vector without taking ownership of it, you can use the `iter()` method to create an iterator over the vector’s elements, and then call `join()` on the iterator instead. Here’s an example:
let my_vec = vec!["hello", "world"];
let joined_string = my_vec.iter().join(", ");
println!("{}", joined_string); // prints "hello, world"
In this example, we call `iter()` on `my_vec` to create an iterator over its elements, and then call `join()` on the iterator to concatenate the elements into a string. The resulting string is stored in `joined_string` and printed to the console.
With Rust’s `join()` method, you can easily concatenate the elements of a vector into a single string, just like you would with JavaScript’s `Array.join()` method.
Equivalent of Javascript Array join in Rust
In conclusion, Rust provides a powerful and efficient way to work with arrays through its standard library. The equivalent of the Javascript Array join function in Rust is the `join` method of the `Vec` type. This method allows us to concatenate the elements of a vector into a single string, using a specified separator. By using Rust’s `join` method, we can easily manipulate and format arrays of data in our Rust programs. This can be particularly useful when working with large datasets or when we need to output data in a specific format. Overall, Rust’s `join` method is a great example of how Rust’s standard library provides developers with powerful tools to work with arrays and other data structures. With its focus on performance and safety, Rust is a great language for building high-performance applications that require efficient data manipulation.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |