The JavaScript Array lastIndexOf function is used to find the last occurrence of a specified element in an array. It searches the array from the end to the beginning and returns the index of the last occurrence of the specified element. If the element is not found in the array, it returns -1. The lastIndexOf function can also take an optional second parameter that specifies the starting index for the search. This function is useful when you need to find the last occurrence of an element in an array, especially when the array contains duplicate elements. Keep reading below to learn how to Javascript Array lastIndexOf in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
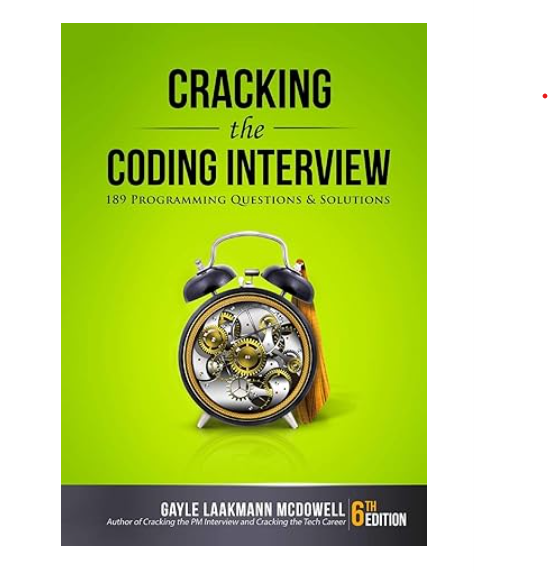
Javascript Array lastIndexOf in C# With Example Code
JavaScript’s `lastIndexOf` method is a useful tool for finding the last occurrence of a specified element in an array. While C# doesn’t have a built-in `lastIndexOf` method for arrays, we can easily create our own implementation.
To create a `lastIndexOf` method in C#, we can loop through the array backwards and return the index of the first occurrence of the specified element. Here’s an example implementation:
public static int LastIndexOf
{
for (int i = array.Length - 1; i >= 0; i--)
{
if (EqualityComparer
{
return i;
}
}
return -1;
}
In this implementation, we use the `EqualityComparer` class to compare the elements in the array to the specified element. This allows us to compare elements of any type, as long as they implement the `IEquatable` interface.
To use this method, simply call it with the array and element you want to search for:
int[] numbers = { 1, 2, 3, 4, 5, 4 };
int lastIndex = LastIndexOf(numbers, 4); // returns 5
With this `lastIndexOf` method, you can easily find the last occurrence of an element in a C# array.
Equivalent of Javascript Array lastIndexOf in C#
In conclusion, the equivalent of the Javascript Array lastIndexOf function in C# is the LastIndexOf method of the List class. This method allows us to search for the last occurrence of a specified element in a List and returns its index. It also provides an overload that allows us to specify the starting index of the search. While the syntax and implementation may differ between the two languages, the functionality remains the same. Both methods are useful for finding the last occurrence of an element in an array or list. By understanding the similarities and differences between these two methods, developers can choose the appropriate one for their specific needs. Whether working with Javascript or C#, having a solid understanding of these methods can help improve the efficiency and effectiveness of your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |