The JavaScript Array lastIndexOf function is used to find the last occurrence of a specified element in an array. It searches the array from the end to the beginning and returns the index of the last occurrence of the specified element. If the element is not found in the array, it returns -1. The lastIndexOf function can also take an optional second parameter that specifies the starting index for the search. This function is useful when you need to find the last occurrence of an element in an array, especially when the array contains duplicate elements. Keep reading below to learn how to Javascript Array lastIndexOf in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
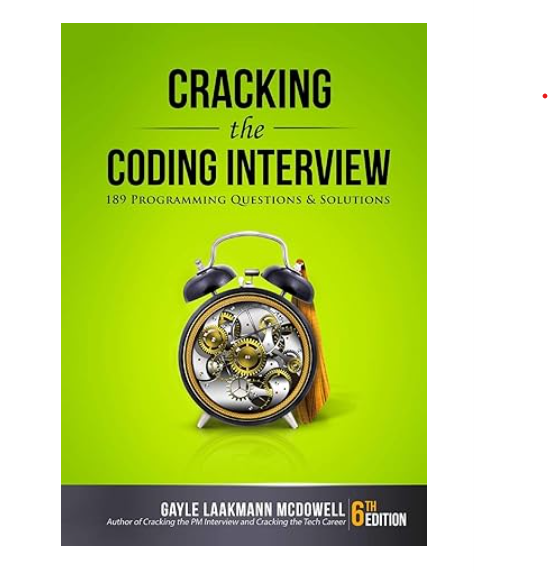
Javascript Array lastIndexOf in C++ With Example Code
JavaScript’s `lastIndexOf()` method is a useful tool for finding the last occurrence of a specified element in an array. However, C++ does not have a built-in function for this. In this blog post, we will explore how to implement the `lastIndexOf()` function in C++.
To implement `lastIndexOf()` in C++, we can use a loop to iterate through the array from the end to the beginning. We can then check each element to see if it matches the specified element. If it does, we return the index of that element.
Here is an example implementation of `lastIndexOf()` in C++:
int lastIndexOf(int arr[], int n, int x) {
for (int i = n - 1; i >= 0; i--) {
if (arr[i] == x) {
return i;
}
}
return -1;
}
In this implementation, `arr` is the array we want to search, `n` is the length of the array, and `x` is the element we want to find the last occurrence of. We start the loop at the end of the array (`n – 1`) and iterate backwards until we reach the beginning (`0`). If we find an element that matches `x`, we return its index. If we reach the end of the loop without finding a match, we return `-1`.
To use this function, we can simply call it with the appropriate arguments:
int arr[] = {1, 2, 3, 4, 5, 4};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 4;
int lastIndex = lastIndexOf(arr, n, x);
In this example, we have an array `arr` with six elements, and we want to find the last occurrence of the number `4`. We pass `arr`, `n`, and `x` to the `lastIndexOf()` function, and it returns the index of the last occurrence of `4`, which is `5`.
In conclusion, while C++ does not have a built-in `lastIndexOf()` function like JavaScript, we can easily implement our own using a loop. This function can be useful in a variety of situations where we need to find the last occurrence of an element in an array.
Equivalent of Javascript Array lastIndexOf in C++
In conclusion, the equivalent of the Javascript Array lastIndexOf function in C++ is the std::find_end function. This function searches for the last occurrence of a given value in a range of elements in a C++ container. It returns an iterator pointing to the last occurrence of the value, or the end iterator if the value is not found. While the syntax and usage of the std::find_end function may differ from the Javascript Array lastIndexOf function, they both serve the same purpose of finding the last occurrence of a value in an array or container. By understanding the similarities and differences between these functions, developers can choose the appropriate tool for their specific programming needs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |