The JavaScript Array lastIndexOf function is used to find the last occurrence of a specified element in an array. It searches the array from right to left and returns the index of the last occurrence of the specified element. If the element is not found in the array, it returns -1. The lastIndexOf function can also take an optional second parameter that specifies the starting index for the search. This function is useful when you need to find the last occurrence of an element in an array, especially when the array contains duplicate elements. Keep reading below to learn how to Javascript Array lastIndexOf in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
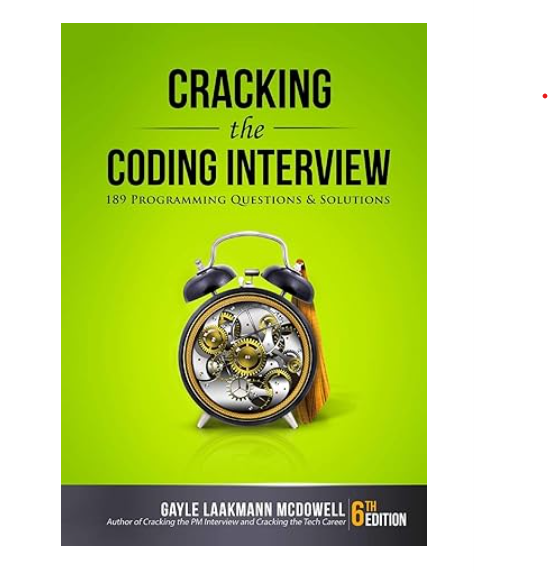
Javascript Array lastIndexOf in Rust With Example Code
JavaScript developers who are transitioning to Rust may find themselves looking for an equivalent to the `lastIndexOf` method for arrays. In JavaScript, `lastIndexOf` returns the index of the last occurrence of a specified element in an array. Rust provides a similar method for vectors, which are Rust’s equivalent to arrays.
To find the last index of an element in a vector in Rust, you can use the `iter().rposition()` method. This method returns an `Option
Here’s an example of how to use `iter().rposition()` to find the last index of an element in a vector:
let vec = vec![1, 2, 3, 4, 5, 4, 3, 2, 1];
let last_index = vec.iter().rposition(|&x| x == 4);
match last_index {
Some(index) => println!("Last index of 4: {}", index),
None => println!("4 not found in vector"),
}
In this example, we create a vector with some integers and then use `iter().rposition()` to find the last index of the number 4. The `|&x| x == 4` closure is used as the predicate to check if an element is equal to 4. The `match` statement is used to handle the `Option
By using `iter().rposition()` in Rust, you can easily find the last index of an element in a vector, which is similar to the functionality provided by `lastIndexOf` in JavaScript.
Equivalent of Javascript Array lastIndexOf in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to work with arrays through its built-in data structure, the Vec. And with the lastIndexOf function, Rust developers can easily search for the last occurrence of a specific element in an array. This function is similar to the equivalent JavaScript Array lastIndexOf function, but with the added benefits of Rust’s strong type system and memory safety guarantees. By leveraging Rust’s array manipulation capabilities, developers can create high-performance and reliable applications that can handle large amounts of data with ease. Overall, the lastIndexOf function is just one example of the many powerful tools that Rust provides for working with arrays, making it a great choice for any developer looking to build robust and efficient applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |