The JavaScript Array map function is a built-in method that allows you to create a new array by applying a function to each element of an existing array. The map function takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The map function returns a new array with the results of the callback function applied to each element of the original array. This is a useful function for transforming data in an array without modifying the original array. Keep reading below to learn how to Javascript Array map in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
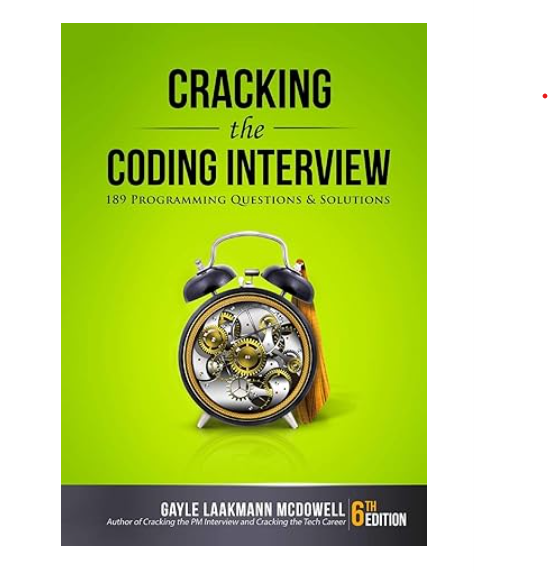
Javascript Array map in C# With Example Code
JavaScript developers are familiar with the Array.map()
method, which creates a new array with the results of calling a provided function on every element in the calling array. This method is useful for transforming data in an array without modifying the original array.
But did you know that you can achieve the same functionality in C# using the Select()
method?
The Select()
method is an extension method provided by LINQ (Language Integrated Query) in C#. It operates on any collection that implements the IEnumerable
interface, including arrays. The method takes a lambda expression as an argument, which is used to transform each element in the collection.
Here’s an example of using Select()
to transform an array of integers:
int[] numbers = { 1, 2, 3, 4, 5 };
var squaredNumbers = numbers.Select(x => x * x).ToArray();
In this example, the lambda expression x => x * x
is used to square each element in the numbers
array. The resulting array, squaredNumbers
, contains the squared values of the original array.
Just like Array.map()
, Select()
does not modify the original array. Instead, it returns a new array with the transformed values.
So next time you need to transform data in a C# array, remember that you can use the Select()
method to achieve the same functionality as Array.map()
in JavaScript.
Equivalent of Javascript Array map in C#
In conclusion, the equivalent of the Javascript Array map function in C# is the LINQ Select method. Both functions allow developers to transform each element of an array or collection into a new value based on a provided function. While the syntax and implementation may differ, the core functionality remains the same. By utilizing the Select method in C#, developers can easily manipulate and transform data in their applications, making it a powerful tool for any developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |