The JavaScript Array map function is a built-in method that allows you to create a new array by applying a function to each element of an existing array. The map function takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The map function returns a new array with the results of the callback function applied to each element of the original array. This is a useful function for transforming data in an array without modifying the original array. Keep reading below to learn how to Javascript Array map in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
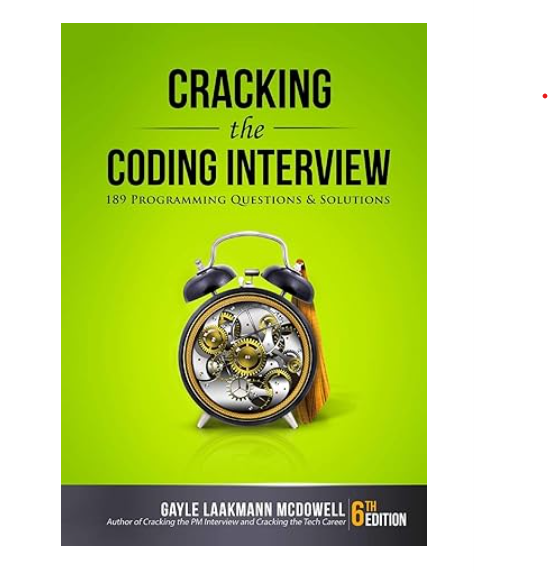
Javascript Array map in C++ With Example Code
JavaScript Array map is a useful method that allows you to apply a function to each element of an array and return a new array with the results. While C++ does not have a built-in map function for arrays, you can easily create your own implementation using the standard library.
To create a map function in C++, you can use the `std::transform` algorithm. This algorithm applies a function to each element of a range and stores the results in another range. Here’s an example of how you can use `std::transform` to create a map function:
#include
#include
#include
template
std::vector
std::vector
std::transform(input.begin(), input.end(), output.begin(), func);
return output;
}
int main() {
std::vector
auto output = map(input, [](int x) { return x * 2; });
for (auto x : output) {
std::cout << x << " ";
}
std::cout << std::endl;
return 0;
}
In this example, we define a `map` function that takes an input vector and a function as arguments. The function applies the given function to each element of the input vector using `std::transform` and returns a new vector with the results.
We then use this `map` function to double each element of an input vector and print the resulting vector.
While C++ does not have a built-in map function for arrays like JavaScript, you can easily create your own implementation using the standard library. The `std::transform` algorithm is a useful tool for creating map functions in C++.
Equivalent of Javascript Array map in C++
In conclusion, the equivalent of the Javascript Array map function in C++ is the transform function. Both functions allow for the transformation of elements in an array or vector without modifying the original data structure. The transform function in C++ is a powerful tool that can be used to simplify code and improve performance by avoiding unnecessary loops and temporary variables. By understanding the similarities and differences between these two functions, developers can leverage the strengths of each language to create efficient and effective code. Whether you are a seasoned programmer or just starting out, the transform function in C++ is a valuable tool to have in your arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |