The JavaScript Array map function is a built-in method that allows you to create a new array by applying a function to each element of an existing array. The map function takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The map function returns a new array with the results of the callback function applied to each element of the original array. This is a useful function for transforming data in an array without modifying the original array. Keep reading below to learn how to Javascript Array map in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
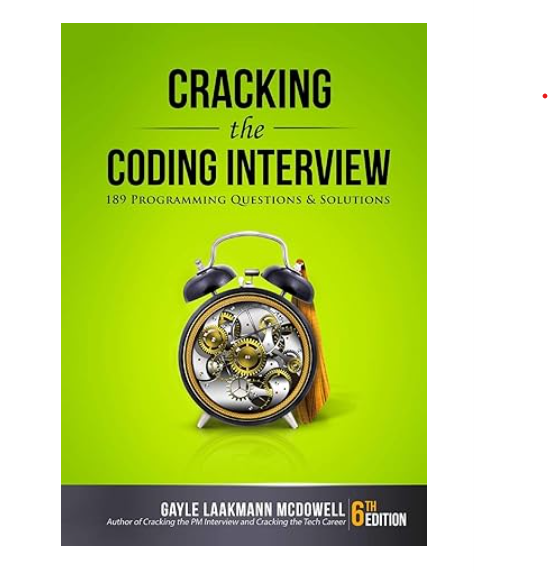
Javascript Array map in Go With Example Code
JavaScript’s `Array.map()` method is a powerful tool for transforming arrays. But what if you’re working in Go? Fortunately, Go has a similar function called `map`, which can be used to achieve the same result.
The `map` function in Go takes two arguments: a function that takes an element of the array as input and returns a new value, and the array to be transformed. The function is applied to each element of the array, and the resulting values are collected into a new array.
Here’s an example of how to use `map` in Go:
func double(x int) int {
return x * 2
}
func main() {
nums := []int{1, 2, 3, 4, 5}
doubled := map(double, nums)
fmt.Println(doubled) // Output: [2 4 6 8 10]
}
In this example, we define a function called `double` that takes an integer and returns its double. We then create an array of integers called `nums` and pass it, along with the `double` function, to the `map` function. The resulting array, `doubled`, contains the doubled values of each element in `nums`.
Using the `map` function in Go can be a powerful way to transform arrays. Whether you’re working with numbers, strings, or any other type of data, `map` can help you quickly and easily transform your data to meet your needs.
Equivalent of Javascript Array map in Go
In conclusion, the equivalent of the Javascript Array map function in Go is the “map” function provided by the language’s built-in “strings” package. This function allows developers to apply a given function to each element of a string array and return a new array with the modified values. While the syntax and usage of the Go map function may differ slightly from its Javascript counterpart, it provides a similar functionality and can be a powerful tool for manipulating string arrays in Go. By leveraging the map function, developers can write more concise and efficient code, making it easier to work with large datasets and complex algorithms. Overall, the Go map function is a valuable addition to any developer’s toolkit and can help streamline the development process for a wide range of applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |