The JavaScript Array map function is a built-in method that allows you to create a new array by applying a function to each element of an existing array. The map function takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The map function returns a new array with the results of the callback function applied to each element of the original array. This is a useful function for transforming data in an array without modifying the original array. Keep reading below to learn how to Javascript Array map in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
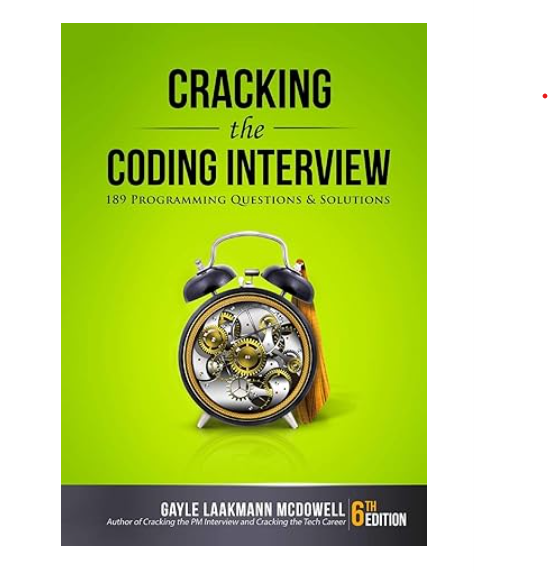
Javascript Array map in Java With Example Code
JavaScript Array map is a method that creates a new array with the results of calling a provided function on every element in the calling array. This method is useful when you want to transform an array into a new array with the same number of elements, but with each element transformed in some way.
In Java, you can use the Stream API to achieve the same functionality as the JavaScript Array map method. The Stream API provides a map() method that can be used to transform the elements of a stream.
Here’s an example of how to use the map() method in Java:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25]
In this example, we first create a list of integers using the Arrays.asList() method. We then create a new stream from this list using the stream() method. We use the map() method to transform each element of the stream by squaring it. Finally, we collect the transformed elements into a new list using the collect() method.
The output of this code will be a list of integers where each element is the square of the corresponding element in the original list.
In conclusion, while Java doesn’t have a built-in method that is exactly equivalent to JavaScript’s Array map method, the Stream API provides a similar functionality that can be used to achieve the same result.
Equivalent of Javascript Array map in Java
In conclusion, the equivalent Java function for the Javascript Array map function is the Stream API’s map() method. This method allows developers to transform the elements of a collection into a new stream of elements based on a given function. The map() method is a powerful tool for manipulating collections in Java, and it can be used in a variety of scenarios to simplify code and improve performance. By understanding the similarities and differences between the Javascript Array map function and the Java Stream API’s map() method, developers can leverage the full power of Java to create efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |