The JavaScript Array map function is a built-in method that allows you to create a new array by applying a function to each element of an existing array. The map function takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The map function returns a new array with the results of the callback function applied to each element of the original array. This is a useful function for transforming data in an array without modifying the original array. Keep reading below to learn how to Javascript Array map in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
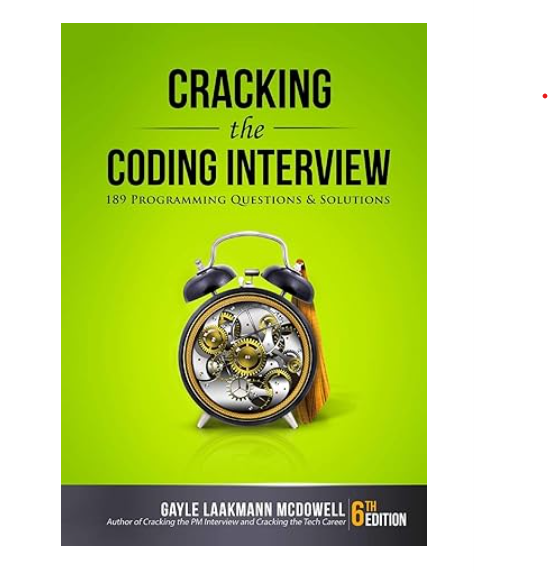
Javascript Array map in Kotlin With Example Code
JavaScript Array map is a useful method that allows you to create a new array by applying a function to each element of an existing array. Kotlin, being a language that is interoperable with JavaScript, also provides a similar method for arrays. In this blog post, we will explore how to use the Kotlin Array map method.
To use the map method in Kotlin, you first need to create an array. Here is an example of how to create an array of integers:
val numbers = arrayOf(1, 2, 3, 4, 5)
Once you have an array, you can use the map method to create a new array by applying a function to each element of the original array. Here is an example of how to use the map method to create a new array of the squares of the elements in the original array:
val squares = numbers.map { it * it }
In this example, we are using a lambda expression to define the function that we want to apply to each element of the original array. The lambda expression takes a single parameter, which represents the current element of the array, and returns the square of that element.
The map method returns a new array that contains the results of applying the function to each element of the original array. In this case, the squares array will contain the values [1, 4, 9, 16, 25].
You can also use the map method with arrays of other types, such as strings or objects. Here is an example of how to use the map method with an array of strings:
val names = arrayOf("Alice", "Bob", "Charlie")
val lengths = names.map { it.length }
In this example, we are using the map method to create a new array that contains the lengths of the strings in the original array. The lengths array will contain the values [5, 3, 7].
In conclusion, the Kotlin Array map method is a powerful tool that allows you to create new arrays by applying functions to the elements of existing arrays. By using lambda expressions, you can easily define the functions that you want to apply, making it easy to manipulate arrays in Kotlin.
Equivalent of Javascript Array map in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to manipulate arrays using the `map` function. This function allows developers to easily transform the elements of an array into a new array, without the need for complex loops or conditional statements. By using the `map` function, developers can write cleaner and more concise code, which is easier to read and maintain. Additionally, Kotlin’s type inference system ensures that the resulting array is of the correct type, which helps to prevent errors and improve code reliability. Overall, the `map` function is a valuable tool for any Kotlin developer who needs to work with arrays, and it is a great example of how Kotlin’s modern features can simplify and streamline the development process.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |