The JavaScript Array map function is a built-in method that allows you to create a new array by applying a function to each element of an existing array. The map function takes a callback function as an argument, which is executed for each element in the array. The callback function can take up to three arguments: the current element being processed, the index of the current element, and the array being processed. The map function returns a new array with the results of the callback function applied to each element of the original array. This is a useful function for transforming data in an array without modifying the original array. Keep reading below to learn how to Javascript Array map in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
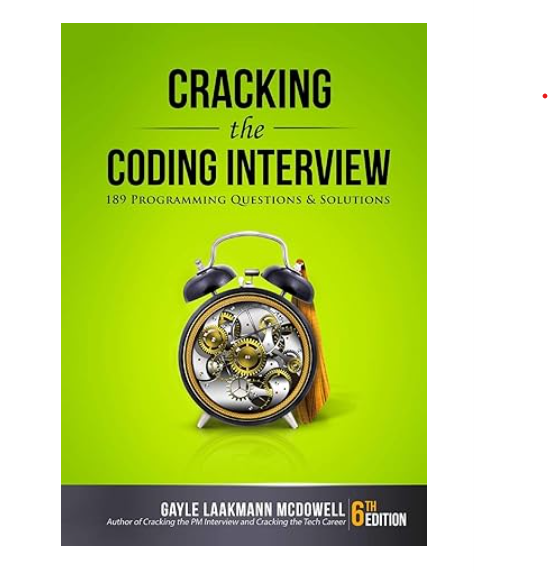
Javascript Array map in Rust With Example Code
JavaScript developers who are familiar with Rust may be interested in using the Rust programming language to map arrays. Rust is a systems programming language that is known for its speed, safety, and concurrency. In this blog post, we will explore how to use Rust to map arrays.
To get started, we need to create a new Rust project. We can do this by running the following command in our terminal:
cargo new rust-array-map
This will create a new Rust project called “rust-array-map”. Next, we need to add the “rayon” crate to our project. Rayon is a data-parallelism library for Rust that makes it easy to parallelize operations on arrays. We can add the “rayon” crate to our project by adding the following line to our “Cargo.toml” file:
rayon = "1.5.0"
Now that we have set up our project, we can start writing our code. Let’s create a new Rust file called “main.rs” in our project’s “src” directory. In this file, we will define a function called “map_array” that takes an array of integers and a closure as arguments. The closure will be used to map each element in the array to a new value.
fn map_array(array: &[i32], f: impl Fn(i32) -> i32) -> Vec
array.par_iter().map(|&x| f(x)).collect()
}
In this code, we are using the “par_iter” method from the “rayon” crate to parallelize the mapping operation. This will make our code run faster on multi-core CPUs.
Now, let’s test our “map_array” function by creating an array of integers and mapping it using a closure that doubles each element:
fn main() {
let array = vec![1, 2, 3, 4, 5];
let doubled_array = map_array(&array, |x| x * 2);
println!("{:?}", doubled_array);
}
When we run this code, we should see the following output:
[2, 4, 6, 8, 10]
This output confirms that our “map_array” function is working correctly.
In conclusion, Rust is a powerful programming language that can be used to map arrays in a fast and safe way. By using the “rayon” crate, we can easily parallelize our code and take advantage of multi-core CPUs. If you are a JavaScript developer who is interested in Rust, we encourage you to give it a try!
Equivalent of Javascript Array map in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate arrays using the `map` function. This function allows developers to apply a transformation to each element of an array and return a new array with the transformed values. The equivalent of the Javascript Array map function in Rust is the `iter()` method, which returns an iterator that can be used with the `map()` method to achieve the same functionality. With Rust’s strong type system and memory safety, developers can confidently use the `map` function to manipulate arrays without worrying about common errors such as null pointer exceptions or memory leaks. Overall, the `map` function in Rust is a valuable tool for developers looking to efficiently manipulate arrays in their programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |