The reduce() function in JavaScript is used to reduce an array of values to a single value. It takes a callback function as its argument, which is executed on each element of the array. The callback function takes two arguments, the accumulator and the current value. The accumulator is the value that is returned after each iteration of the callback function, and the current value is the value of the current element being processed. The reduce() function can also take an optional initial value for the accumulator. The final value of the accumulator is returned after all elements have been processed. The reduce() function is commonly used for operations such as summing up the values in an array or finding the maximum or minimum value. Keep reading below to learn how to Javascript Array reduce in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
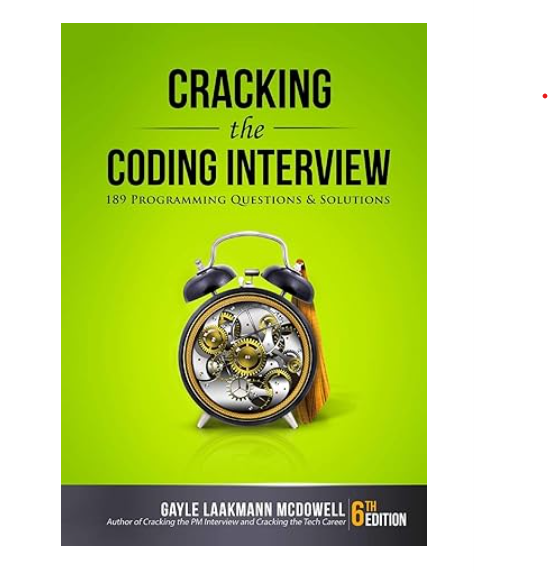
Javascript Array reduce in Bash With Example Code
JavaScript’s `reduce()` method is a powerful tool for working with arrays. But did you know that you can use it in Bash as well? In this post, we’ll explore how to use `reduce()` in Bash to manipulate arrays.
First, let’s review what `reduce()` does. It takes an array and a callback function as arguments. The callback function takes two parameters: an accumulator and the current value of the array. The callback function performs some operation on these two values and returns the result, which becomes the new value of the accumulator. The `reduce()` method then moves on to the next value in the array and repeats the process until it has gone through all the values in the array.
To use `reduce()` in Bash, we’ll need to define our array and our callback function. Here’s an example:
#!/bin/bash
# Define the array
my_array=(1 2 3 4 5)
# Define the callback function
function sum {
echo $(($1 + $2))
}
# Use reduce to sum the array
result=$(echo ${my_array[@]} | tr ' ' '\n' | awk '{sum+=$1} END {print sum}')
echo $result
In this example, we define an array called `my_array` with the values 1 through 5. We also define a callback function called `sum` that takes two parameters and returns their sum. Finally, we use the `reduce()` method to sum the values in the array.
To use `reduce()` in Bash, we need to convert our array into a format that can be processed by the `reduce()` method. We do this by using the `tr` command to replace spaces with newlines, and then using `awk` to sum the values.
In this example, the result of the `reduce()` method is stored in the `result` variable, which we then print to the console.
Using `reduce()` in Bash can be a powerful tool for manipulating arrays. By defining a callback function and using the `reduce()` method, we can perform complex operations on arrays with ease.
Equivalent of Javascript Array reduce in Bash
In conclusion, the equivalent of the Javascript Array reduce function in Bash is a powerful tool that can simplify complex data manipulation tasks. By using the `awk` command and its built-in `reduce` function, Bash users can easily perform calculations on arrays of data, filter out unwanted elements, and transform data into new formats. While the syntax may be slightly different from Javascript, the core functionality remains the same, making it a valuable addition to any Bash programmer’s toolkit. Whether you’re working with large datasets or just need to perform a quick calculation, the Bash reduce function is a versatile and efficient solution that can help you get the job done.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |