The reduce() function in JavaScript is used to reduce an array of values to a single value. It takes a callback function as its argument, which is executed on each element of the array. The callback function takes two arguments, the accumulator and the current value. The accumulator is the value that is returned after each iteration, and the current value is the value of the current element being processed. The reduce() function can also take an optional initial value as its second argument, which is used as the initial value of the accumulator. The final value of the accumulator is returned after all elements have been processed. The reduce() function is commonly used for operations such as summing up the values in an array or finding the maximum or minimum value. Keep reading below to learn how to Javascript Array reduce in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
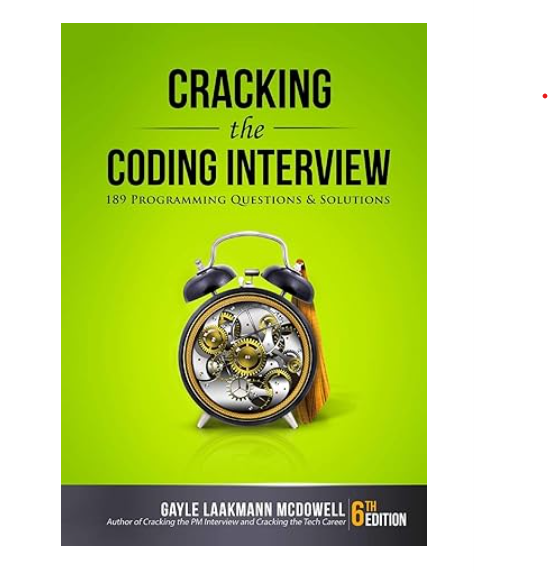
Javascript Array reduce in Kotlin With Example Code
JavaScript’s `reduce()` method is a powerful tool for working with arrays. It allows you to iterate over an array and accumulate a single value based on the elements in the array. Kotlin also has a similar method called `reduce()` that works in a similar way. In this blog post, we’ll explore how to use `reduce()` in Kotlin to work with arrays.
To use `reduce()` in Kotlin, you first need an array to work with. Here’s an example array:
val numbers = arrayOf(1, 2, 3, 4, 5)
Now, let’s say we want to use `reduce()` to find the sum of all the numbers in the array. We can do this by passing a lambda function to `reduce()` that takes two arguments: the accumulated value and the current element in the array. Here’s what that looks like:
val sum = numbers.reduce { acc, num -> acc + num }
In this example, `acc` is the accumulated value and `num` is the current element in the array. The lambda function simply adds the current element to the accumulated value and returns the result. The result of this code will be `15`, which is the sum of all the numbers in the array.
You can also use `reduce()` to perform other operations on arrays, such as finding the maximum or minimum value. Here’s an example of how to find the maximum value in an array:
val max = numbers.reduce { acc, num -> if (acc > num) acc else num }
In this example, the lambda function compares the accumulated value to the current element and returns the larger of the two. The result of this code will be `5`, which is the maximum value in the array.
In conclusion, `reduce()` is a powerful method for working with arrays in Kotlin. By passing a lambda function to `reduce()`, you can perform a wide variety of operations on arrays, from finding the sum to finding the maximum or minimum value.
Equivalent of Javascript Array reduce in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to manipulate arrays using the `reduce` function. This function allows developers to perform complex operations on arrays with ease, reducing the amount of code needed to achieve the desired result. By using the `reduce` function, developers can simplify their code and make it more readable, while also improving the performance of their applications. Whether you are a seasoned Kotlin developer or just starting out, the `reduce` function is a valuable tool to have in your arsenal. So, give it a try and see how it can help you streamline your array operations in Kotlin.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |