The reduce() function in JavaScript is used to reduce an array of values to a single value. It takes a callback function as its argument, which is executed on each element of the array. The callback function takes two arguments, the accumulator and the current value. The accumulator is the value that is returned after each iteration of the callback function, and the current value is the value of the current element being processed. The reduce() function can also take an optional initial value for the accumulator. The final value of the accumulator is returned after all elements have been processed. The reduce() function is commonly used for operations such as summing up the values in an array or finding the maximum or minimum value. Keep reading below to learn how to Javascript Array reduce in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
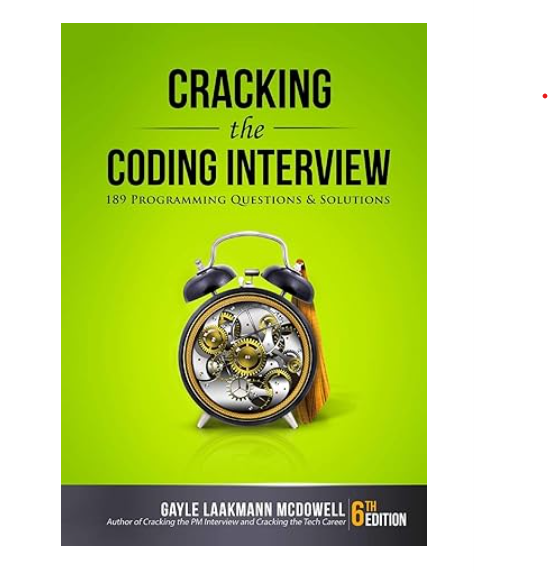
Javascript Array reduce in Rust With Example Code
JavaScript developers are likely familiar with the `reduce()` method on arrays, which allows for the reduction of an array to a single value. Rust developers can achieve similar functionality with the `fold()` method on iterators.
To use `fold()` on an array in Rust, we first need to convert the array to an iterator using the `iter()` method. Then, we can call `fold()` on the iterator and pass in an initial value and a closure that takes two arguments: an accumulator and the current element in the iteration.
Here’s an example of using `fold()` on an array of integers to calculate the sum:
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.iter().fold(0, |acc, x| acc + x);
println!("The sum is {}", sum);
In this example, we start with an initial value of 0 and add each element in the array to the accumulator. The final value of the accumulator is returned as the result of the `fold()` method.
`fold()` can also be used to perform other operations on arrays, such as finding the maximum or minimum value, or concatenating strings.
Overall, `fold()` provides a powerful tool for working with arrays in Rust, allowing for efficient and concise code.
Equivalent of Javascript Array reduce in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to work with arrays through its equivalent of the Javascript Array reduce function. With Rust’s functional programming features and its ability to handle complex data structures, developers can easily manipulate arrays and perform various operations on them using the reduce function. The reduce function in Rust is a great tool for simplifying code and improving performance, making it an essential feature for any Rust developer working with arrays. Overall, the Rust equivalent of the Javascript Array reduce function is a valuable addition to the language and a great tool for developers looking to streamline their code and improve their productivity.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |