The reduce() function in JavaScript is used to reduce an array of values to a single value. It takes a callback function as its argument, which is executed on each element of the array. The callback function takes two arguments, the accumulator and the current value. The accumulator is the value that is returned after each iteration, and the current value is the value of the current element being processed. The reduce() function can also take an optional initial value as its second argument, which is used as the initial value of the accumulator. The final value of the accumulator is returned after all elements have been processed. The reduce() function is commonly used for operations such as summing up the values in an array or finding the maximum or minimum value. Keep reading below to learn how to Javascript Array reduce in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
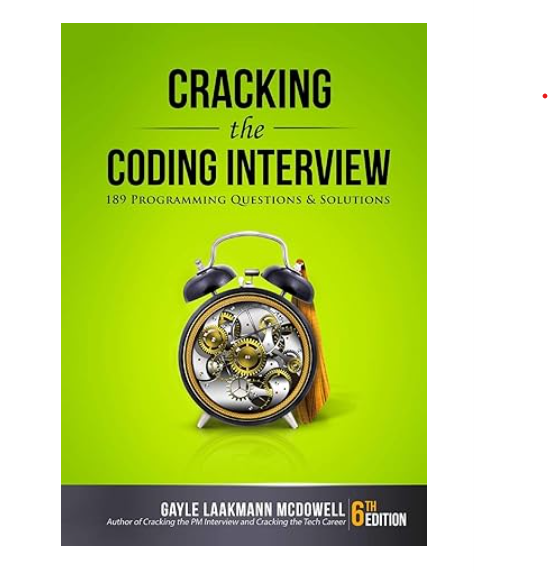
Javascript Array reduce in TypeScript With Example Code
JavaScript arrays are a fundamental data structure in the language. They allow developers to store and manipulate collections of data in a variety of ways. One of the most powerful methods available for working with arrays is the `reduce()` method. In this blog post, we will explore how to use the `reduce()` method in TypeScript.
The `reduce()` method is used to iterate over an array and accumulate a single value based on the elements in the array. The method takes a callback function as its first argument, which is executed on each element in the array. The callback function takes two arguments: an accumulator and the current element being processed. The accumulator is the value that is returned and passed to the next iteration of the callback function.
Here is an example of using the `reduce()` method to calculate the sum of an array of numbers:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(sum); // Output: 15
In this example, we start with an array of numbers and initialize the accumulator to 0. The callback function takes the accumulator and adds the current value to it, returning the new value. The `reduce()` method then passes this new value as the accumulator to the next iteration of the callback function.
It is important to note that the `reduce()` method returns the final value of the accumulator. In the example above, the final value of the accumulator is 15, which is the sum of all the numbers in the array.
In TypeScript, we can provide type annotations for the accumulator and current value parameters in the callback function. This can help catch type errors at compile time and make our code more robust. Here is an example of using type annotations with the `reduce()` method:
interface Person {
name: string;
age: number;
}
const people: Person[] = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 },
];
const totalAge = people.reduce((accumulator: number, currentValue: Person) => {
return accumulator + currentValue.age;
}, 0);
console.log(totalAge); // Output: 90
In this example, we have an array of `Person` objects and we want to calculate the total age of all the people in the array. We provide type annotations for the accumulator and current value parameters in the callback function to ensure that we are working with the correct types.
In conclusion, the `reduce()` method is a powerful tool for working with arrays in JavaScript and TypeScript. By providing a callback function that accumulates a single value based on the elements in the array, we can perform complex operations on the data in the array. With the addition of type annotations in TypeScript, we can make our code more robust and catch type errors at compile time.
Equivalent of Javascript Array reduce in TypeScript
In conclusion, the TypeScript language provides a powerful and efficient way to work with arrays through its built-in Array reduce function. This function allows developers to easily manipulate and transform arrays, while also providing type safety and error checking. By using the reduce function in TypeScript, developers can write cleaner and more concise code, while also improving the overall performance of their applications. Whether you are a seasoned developer or just starting out with TypeScript, the Array reduce function is a valuable tool that can help you take your coding skills to the next level.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |