The reduceRight() function in JavaScript is used to apply a function to each element of an array from right to left, reducing the array to a single value. It takes two arguments, a callback function and an optional initial value. The callback function takes four arguments, the accumulator, the current value, the current index, and the array itself. The reduceRight() function starts with the last element of the array and iterates through each element, applying the callback function to the accumulator and the current value. The result of each iteration is passed as the accumulator to the next iteration until all elements have been processed, resulting in a single value. If an initial value is provided, it is used as the initial accumulator value, otherwise, the last element of the array is used as the initial accumulator value. Keep reading below to learn how to Javascript Array reduceRight in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
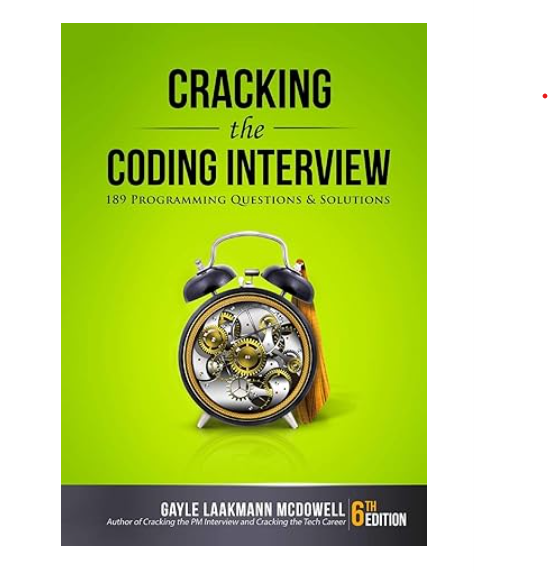
Javascript Array reduceRight in Kotlin With Example Code
JavaScript’s `reduceRight()` method is a powerful tool for working with arrays. It allows you to iterate over an array from right to left, applying a function to each element and accumulating a result. In Kotlin, you can use the `reduceRight()` method to achieve the same functionality.
To use `reduceRight()` in Kotlin, you first need to define an array. Here’s an example:
val numbers = arrayOf(1, 2, 3, 4, 5)
Next, you can call the `reduceRight()` method on the array. This method takes a function as an argument, which will be applied to each element of the array. The function should take two arguments: the accumulator and the current element. Here’s an example:
val sum = numbers.reduceRight { acc, num -> acc + num }
In this example, the `reduceRight()` method is called on the `numbers` array. The function passed to `reduceRight()` takes two arguments: `acc` (the accumulator) and `num` (the current element). The function simply adds the current element to the accumulator and returns the result.
The `reduceRight()` method returns the final value of the accumulator. In this example, the `sum` variable will contain the value `15`, which is the sum of all the elements in the `numbers` array.
Overall, the `reduceRight()` method is a useful tool for working with arrays in Kotlin. It allows you to easily iterate over an array from right to left and accumulate a result along the way.
Equivalent of Javascript Array reduceRight in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to manipulate arrays using the reduceRight function. This function works similarly to its equivalent in JavaScript, allowing developers to perform complex operations on arrays with ease. By using reduceRight, developers can iterate through an array from right to left, reducing the array to a single value based on a given operation. This can be incredibly useful for a wide range of applications, from simple calculations to more complex data processing tasks. Overall, the reduceRight function in Kotlin is a valuable tool for any developer looking to work with arrays in a more efficient and effective way.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |