The reduceRight() function in JavaScript is used to apply a function to each element of an array from right to left, reducing the array to a single value. It takes two arguments, a callback function and an optional initial value. The callback function takes four arguments, the accumulator, the current value, the current index, and the array itself. The reduceRight() function starts with the last element of the array and iterates through each element, applying the callback function to the accumulator and the current value. The result of each iteration is passed as the accumulator to the next iteration until all elements have been processed, resulting in a single value. If an initial value is provided, it is used as the initial accumulator value, otherwise, the last element of the array is used as the initial accumulator value. Keep reading below to learn how to Javascript Array reduceRight in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
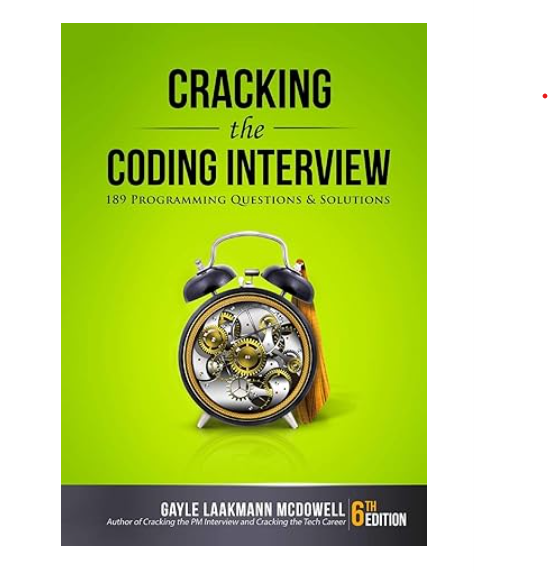
Javascript Array reduceRight in PHP With Example Code
JavaScript’s `reduceRight()` method is a powerful tool for working with arrays. It allows you to iterate over an array from right to left, applying a function to each element and accumulating a result. But what if you’re working in PHP? Fortunately, PHP has a similar method called `array_reduce()` that can accomplish the same task.
To use `array_reduce()` in PHP, you’ll need to pass it an array and a callback function. The callback function should take two arguments: the accumulated value and the current element of the array. It should return the new accumulated value after processing the current element.
Here’s an example of using `array_reduce()` to find the sum of an array of numbers:
$numbers = [1, 2, 3, 4, 5];
$sum = array_reduce($numbers, function($acc, $num) {
return $acc + $num;
}, 0);
echo $sum; // Output: 15
In this example, we start with an array of numbers and an initial value of 0 for the accumulator. The callback function takes the current accumulator value and adds the current number to it, returning the new accumulator value. Finally, we output the sum of the array.
You can also use `array_reduce()` to perform more complex operations on arrays. For example, you could use it to concatenate a list of strings:
$strings = ['hello', 'world', 'how', 'are', 'you'];
$concatenated = array_reduce($strings, function($acc, $str) {
return $acc . ' ' . $str;
}, '');
echo $concatenated; // Output: "hello world how are you"
In this example, we start with an array of strings and an initial value of an empty string for the accumulator. The callback function takes the current accumulator value and concatenates it with the current string, returning the new accumulator value. Finally, we output the concatenated string.
In conclusion, `array_reduce()` is a powerful method in PHP that can accomplish many of the same tasks as JavaScript’s `reduceRight()` method. By passing it an array and a callback function, you can iterate over the array and accumulate a result based on each element.
Equivalent of Javascript Array reduceRight in PHP
In conclusion, the equivalent of the Javascript Array reduceRight function in PHP is the array_reduce function with the optional third parameter set to true. This function allows us to iterate over an array from right to left and apply a callback function to each element, accumulating a single value as a result. By using this function, we can simplify our code and make it more efficient, especially when dealing with large arrays. Overall, the array_reduce function with the true parameter is a powerful tool in PHP that can help us solve complex problems with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |