The reduceRight() function in JavaScript is used to apply a function to each element of an array from right to left, reducing the array to a single value. It takes two arguments, a callback function and an optional initial value. The callback function takes four arguments: the accumulator, the current value, the current index, and the array itself. The accumulator is the value returned by the previous iteration of the callback function, or the initial value if provided. The reduceRight() function is useful for performing operations on an array in reverse order, such as concatenating strings or finding the maximum value. Keep reading below to learn how to Javascript Array reduceRight in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
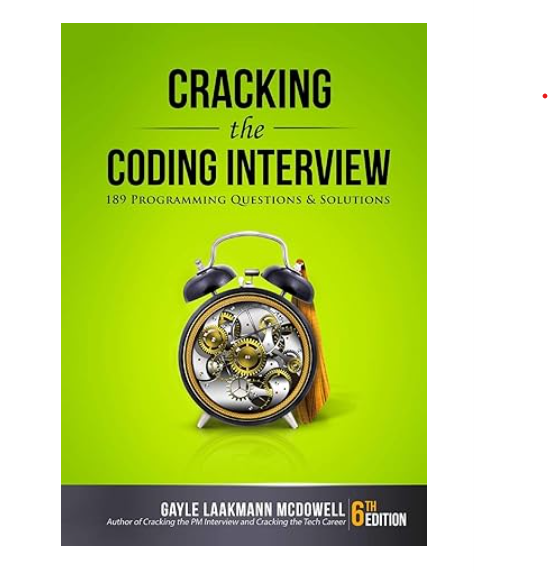
Javascript Array reduceRight in Rust With Example Code
JavaScript developers are likely familiar with the `reduceRight` method on arrays, which applies a function against an accumulator and each value of the array (from right-to-left) to reduce it to a single value. Rust developers can achieve similar functionality with the `fold` method on iterators.
To use `fold` in Rust, we first need to create an iterator from our array. We can do this using the `iter` method on the array. Then, we can call `fold` on the iterator and pass in an initial value for the accumulator and a closure that takes two arguments: the current value and the accumulator. The closure should return the new value of the accumulator.
Here’s an example of using `fold` to find the product of an array of numbers:
let numbers = [1, 2, 3, 4, 5];
let product = numbers.iter().fold(1, |acc, x| acc * x);
println!("Product: {}", product);
In this example, we start with an initial value of 1 for the accumulator and multiply it by each value in the array to get the final product.
By default, `fold` processes the iterator from left-to-right. To process it from right-to-left, we can use the `rev` method on the iterator to reverse it before calling `fold`.
let numbers = [1, 2, 3, 4, 5];
let product = numbers.iter().rev().fold(1, |acc, x| acc * x);
println!("Product (reversed): {}", product);
In this example, we reverse the iterator before calling `fold`, so the values are processed from right-to-left.
While `fold` doesn’t have the same syntax as `reduceRight` in JavaScript, it provides similar functionality and can be used to achieve the same results.
Equivalent of Javascript Array reduceRight in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate arrays using the `reduceRight` function. This function allows developers to iterate over an array from right to left, applying a given function to each element and accumulating the results into a single value. With Rust’s strong type system and memory safety guarantees, developers can write safe and performant code that can handle large arrays with ease. Whether you’re a seasoned Rust developer or just getting started, the `reduceRight` function is a valuable tool to have in your arsenal for working with arrays.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |