The reduceRight() function in JavaScript is used to apply a function to each element of an array from right to left, reducing the array to a single value. It takes two arguments, a callback function and an optional initial value. The callback function takes four arguments, the accumulator, the current value, the current index, and the array itself. The reduceRight() function starts with the last element of the array and iterates through each element, applying the callback function to the accumulator and the current value. The result of each iteration is passed as the accumulator to the next iteration until all elements have been processed, resulting in a single value. If an initial value is provided, it is used as the initial accumulator value, otherwise, the last element of the array is used as the initial accumulator value. Keep reading below to learn how to Javascript Array reduceRight in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
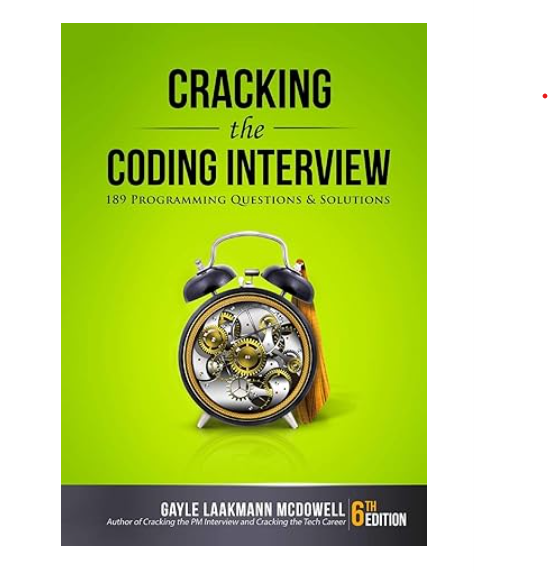
Javascript Array reduceRight in TypeScript With Example Code
JavaScript arrays are a powerful tool for storing and manipulating data. One of the most useful methods available for arrays is the `reduceRight()` method. This method is used to reduce an array to a single value by iterating over the elements of the array from right to left. In this blog post, we will explore how to use the `reduceRight()` method in TypeScript.
To use the `reduceRight()` method in TypeScript, we first need to define an array. Let’s create an array of numbers:
const numbers = [1, 2, 3, 4, 5];
Now that we have an array, we can use the `reduceRight()` method to iterate over the elements of the array from right to left. The `reduceRight()` method takes two arguments: a callback function and an optional initial value. The callback function is called for each element of the array, and it takes four arguments: the previous value, the current value, the current index, and the array itself.
Here is an example of using the `reduceRight()` method to sum the elements of an array:
const sum = numbers.reduceRight((previousValue, currentValue) => {
return previousValue + currentValue;
});
In this example, the `reduceRight()` method is called on the `numbers` array. The callback function takes two arguments: `previousValue` and `currentValue`. The `previousValue` is the accumulated value from the previous iteration, and the `currentValue` is the current element of the array. The callback function returns the sum of the `previousValue` and `currentValue`.
Finally, the `reduceRight()` method returns the final accumulated value, which in this case is the sum of all the elements of the array.
In conclusion, the `reduceRight()` method is a powerful tool for manipulating arrays in TypeScript. By iterating over the elements of an array from right to left, we can easily reduce an array to a single value.
Equivalent of Javascript Array reduceRight in TypeScript
In conclusion, the TypeScript language provides developers with a powerful tool in the form of the Array reduceRight function. This function allows developers to iterate over an array in reverse order and apply a callback function to each element, accumulating a single value as a result. By using the reduceRight function, developers can write more concise and efficient code, reducing the need for complex loops and conditional statements. Additionally, the type safety provided by TypeScript ensures that developers can catch errors early in the development process, leading to more robust and reliable code. Overall, the reduceRight function is a valuable addition to the TypeScript language, and developers should take advantage of its capabilities to improve their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |