The JavaScript Array shift() function is used to remove the first element from an array and return that element. This function modifies the original array by removing the first element and shifting all other elements down by one index. If the array is empty, the shift() function returns undefined. The shift() function is useful when you need to remove the first element of an array and process it separately, such as when implementing a queue data structure. Keep reading below to learn how to Javascript Array shift in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
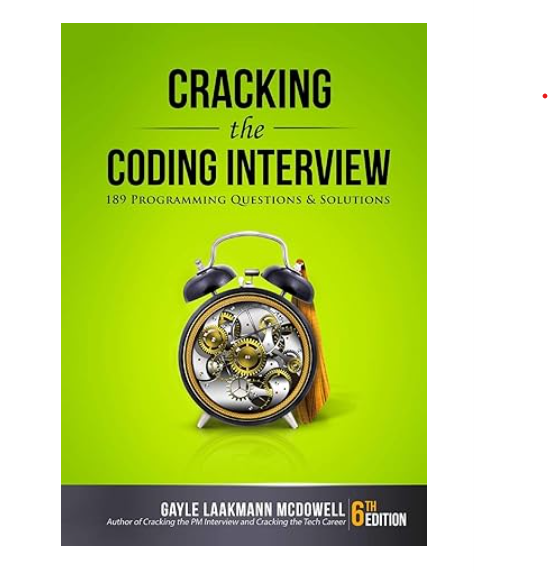
Javascript Array shift in C# With Example Code
JavaScript’s array shift method is a useful tool for removing the first element of an array and returning it. However, if you’re working in C#, you may be wondering how to achieve the same functionality. Fortunately, C# provides a similar method called “Dequeue” that can be used to remove the first element of a queue.
To use Dequeue, you’ll first need to create a Queue object and add elements to it. Here’s an example:
Queue
myQueue.Enqueue("apple");
myQueue.Enqueue("banana");
myQueue.Enqueue("cherry");
In this example, we’ve created a Queue object called “myQueue” and added three elements to it using the Enqueue method.
To remove the first element of the queue and return it, we can use the Dequeue method like this:
string firstElement = myQueue.Dequeue();
In this example, we’ve assigned the value of the first element in the queue to a string variable called “firstElement” using the Dequeue method.
It’s important to note that Dequeue will throw an InvalidOperationException if the queue is empty. To avoid this, you can check the Count property of the queue before calling Dequeue:
if (myQueue.Count > 0)
{
string firstElement = myQueue.Dequeue();
}
In this example, we’ve added an if statement to check if the queue contains any elements before calling Dequeue.
Using Dequeue in C# is a simple and effective way to achieve the same functionality as JavaScript’s array shift method.
Equivalent of Javascript Array shift in C#
In conclusion, the C# programming language provides a similar functionality to the Javascript Array shift function through the use of the List
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |