The JavaScript Array shift() function is used to remove the first element from an array and return that element. This function modifies the original array by removing the first element and shifting all other elements down by one index. If the array is empty, the shift() function returns undefined. The shift() function is useful when you need to remove the first element of an array and process it separately, such as when implementing a queue data structure. Keep reading below to learn how to Javascript Array shift in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
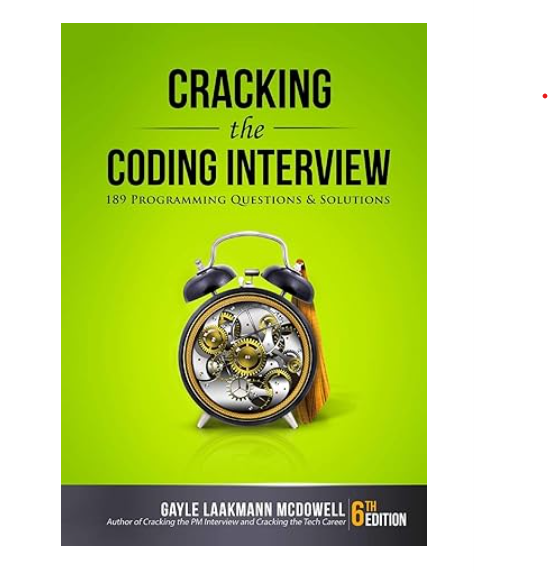
Javascript Array shift in C++ With Example Code
JavaScript Array shift() method is used to remove the first element from an array and returns that removed element. In C++, we can achieve the same functionality using the std::vector container and its erase() method.
To remove the first element from a vector, we can use the erase() method with the iterator pointing to the first element. Here’s an example code snippet:
#include
#include
int main() {
std::vector
vec.erase(vec.begin());
for (auto i : vec) {
std::cout << i << " ";
}
return 0;
}
In the above code, we first create a vector with some elements. Then, we use the erase() method with the iterator pointing to the first element to remove it from the vector. Finally, we iterate over the vector and print its elements to verify that the first element has been removed.
Using the erase() method with the iterator pointing to the first element is equivalent to using the shift() method in JavaScript.
Equivalent of Javascript Array shift in C++
In conclusion, the equivalent C++ function for the Javascript Array shift() function is the std::vector::erase() function. This function removes the first element of a vector and shifts all the remaining elements to the left. It is important to note that the erase() function can be used to remove any element from a vector, not just the first one. Additionally, the erase() function can be used in conjunction with other vector functions to manipulate the contents of a vector in various ways. Overall, the erase() function is a powerful tool for working with vectors in C++, and can be used to achieve similar functionality to the shift() function in Javascript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |