The JavaScript Array shift() function is used to remove the first element from an array and return that element. This function modifies the original array by removing the first element and shifting all other elements down by one index. If the array is empty, the shift() function returns undefined. The shift() function is useful when you need to remove the first element of an array and process it separately, such as when implementing a queue data structure. Keep reading below to learn how to Javascript Array shift in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
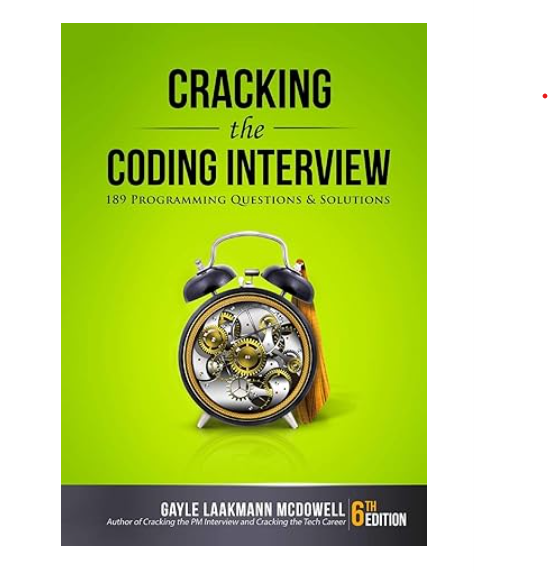
Javascript Array shift in Go With Example Code
JavaScript’s array method `shift()` is used to remove the first element from an array and return that element. In Go, we can achieve the same functionality using slices.
To remove the first element from a slice in Go, we can use the built-in function `copy()` to shift all the elements one position to the left and then resize the slice to exclude the first element.
Here’s an example code snippet that demonstrates how to shift a slice in Go:
func shiftSlice(slice []int) []int {
if len(slice) == 0 {
return slice
}
copy(slice, slice[1:])
return slice[:len(slice)-1]
}
In this example, we first check if the slice is empty. If it is, we simply return the original slice. Otherwise, we use the `copy()` function to shift all the elements one position to the left. Finally, we resize the slice to exclude the first element using the slice operator `[:len(slice)-1]`.
Using this function, we can shift any slice in Go, including slices of different types such as strings or structs.
Equivalent of Javascript Array shift in Go
In conclusion, the equivalent of the Javascript Array shift function in Go is the built-in function `copy()`. While the syntax and usage may differ slightly, both functions serve the same purpose of removing the first element of an array and shifting all remaining elements to the left. It is important to note that the `copy()` function in Go requires the destination slice to have enough capacity to hold all the elements being copied, which may require some additional code to ensure proper functionality. Overall, understanding the similarities and differences between these functions can help developers efficiently manipulate arrays in both Javascript and Go.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |