The JavaScript Array shift() function is used to remove the first element from an array and return that element. This function modifies the original array by removing the first element and shifting all other elements down by one index. If the array is empty, the shift() function returns undefined. The shift() function is useful when you need to remove the first element of an array and process it separately, such as when implementing a queue data structure. Keep reading below to learn how to Javascript Array shift in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
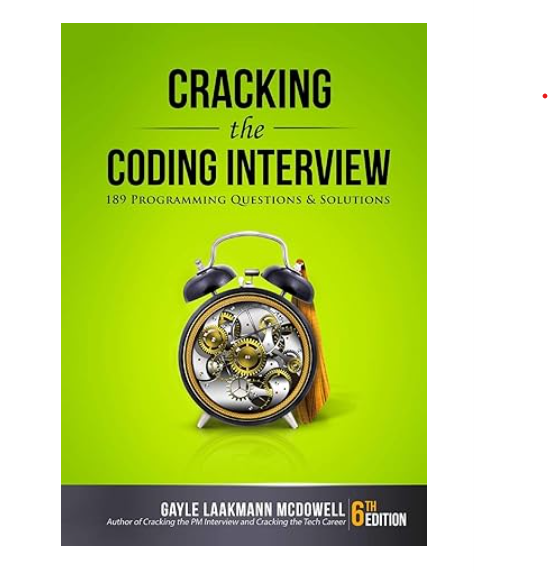
Javascript Array shift in Python With Example Code
JavaScript and Python are two of the most popular programming languages in the world. While they have many similarities, there are also some differences between the two. One of these differences is how they handle arrays. In JavaScript, there is a method called “shift” that allows you to remove the first element of an array and return that element. In Python, there is no built-in method for this, but there are ways to achieve the same result.
To shift an array in JavaScript, you can use the “shift” method. Here is an example:
let myArray = [1, 2, 3, 4, 5];
let firstElement = myArray.shift();
console.log(firstElement); // Output: 1
console.log(myArray); // Output: [2, 3, 4, 5]
In this example, we have an array called “myArray” with five elements. We then use the “shift” method to remove the first element of the array and store it in a variable called “firstElement”. We then log the value of “firstElement” to the console, which should output “1”. Finally, we log the value of “myArray” to the console, which should output “[2, 3, 4, 5]”.
To achieve the same result in Python, you can use slicing. Here is an example:
myList = [1, 2, 3, 4, 5]
firstElement = myList.pop(0)
print(firstElement) # Output: 1
print(myList) # Output: [2, 3, 4, 5]
In this example, we have a list called “myList” with five elements. We then use the “pop” method with an index of 0 to remove the first element of the list and store it in a variable called “firstElement”. We then print the value of “firstElement” to the console, which should output “1”. Finally, we print the value of “myList” to the console, which should output “[2, 3, 4, 5]”.
While the syntax is different between the two languages, the end result is the same. By using the “shift” method in JavaScript or slicing in Python, you can remove the first element of an array or list and return that element.
Equivalent of Javascript Array shift in Python
In conclusion, the equivalent function of the Javascript Array shift() method in Python is the list.pop(0) method. Both methods remove the first element of an array or list and return that element. While the syntax may differ, the functionality remains the same. It is important to note that the shift() method in Javascript modifies the original array, whereas the pop() method in Python returns a new list without the first element. Understanding the similarities and differences between these methods can help developers efficiently manipulate arrays and lists in both languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |