The JavaScript Array shift() function is used to remove the first element from an array and return that element. This function modifies the original array by removing the first element and shifting all other elements down by one index. If the array is empty, the shift() function returns undefined. The shift() function is useful when you need to remove the first element of an array and process it separately, such as when implementing a queue data structure. Keep reading below to learn how to Javascript Array shift in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
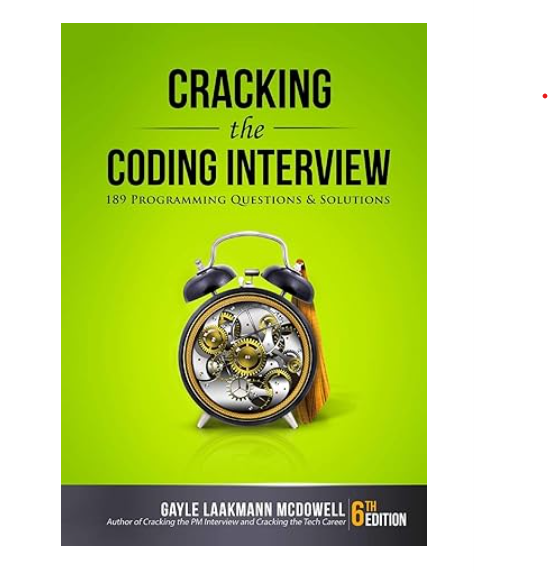
Javascript Array shift in Rust With Example Code
JavaScript’s `Array.shift()` method removes the first element from an array and returns it. Rust provides a similar functionality through its `Vec` type. In this blog post, we will explore how to implement the `Array.shift()` method in Rust.
To start, let’s create a new Rust project and add the following code to the `main.rs` file:
fn main() {
let mut vec = vec![1, 2, 3, 4, 5];
let first = vec.remove(0);
println!("Removed element: {}", first);
println!("Remaining elements: {:?}", vec);
}
In this code, we create a new `Vec` with five elements and then remove the first element using the `remove()` method. The removed element is stored in the `first` variable, and the remaining elements are printed to the console.
The `remove()` method takes an index as an argument and removes the element at that index from the vector. In this case, we pass `0` as the index to remove the first element.
If we run this code, we should see the following output:
Removed element: 1
Remaining elements: [2, 3, 4, 5]
This output indicates that the first element was successfully removed from the vector, and the remaining elements were shifted down by one index.
To make this functionality more like `Array.shift()`, we can wrap it in a function that takes a vector as an argument and returns the removed element. Here’s an example implementation:
fn shift
if vec.is_empty() {
None
} else {
Some(vec.remove(0))
}
}
This function takes a mutable reference to a vector and returns an `Option` that contains the removed element if the vector was not empty, or `None` if the vector was empty.
We can use this function like so:
fn main() {
let mut vec = vec![1, 2, 3, 4, 5];
if let Some(first) = shift(&mut vec) {
println!("Removed element: {}", first);
println!("Remaining elements: {:?}", vec);
} else {
println!("Vector is empty");
}
}
In this code, we call the `shift()` function with a mutable reference to the vector. If the function returns `Some`, we print the removed element and the remaining elements. If the function returns `None`, we print a message indicating that the vector is empty.
With this implementation, we can now use Rust’s `Vec` type to achieve similar functionality to JavaScript’s `Array.shift()` method.
Equivalent of Javascript Array shift in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate arrays with its built-in methods. One such method is the equivalent of the Javascript Array shift function, which allows developers to remove the first element of an array and return it. This method is called `remove` in Rust and can be used to achieve the same functionality as the shift function in Javascript. By using Rust’s array manipulation methods, developers can write efficient and reliable code that can handle large amounts of data with ease. Overall, Rust’s array manipulation capabilities make it a great choice for developers who want to build high-performance applications that require efficient data handling.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |