The JavaScript Array slice function is used to extract a portion of an array and return a new array with the extracted elements. It takes two arguments: the starting index and the ending index (optional). The starting index is the position of the first element to be included in the new array, while the ending index is the position of the last element to be included (not inclusive). If the ending index is not specified, the slice function will extract all elements from the starting index to the end of the array. The original array is not modified by the slice function. Keep reading below to learn how to Javascript Array slice in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
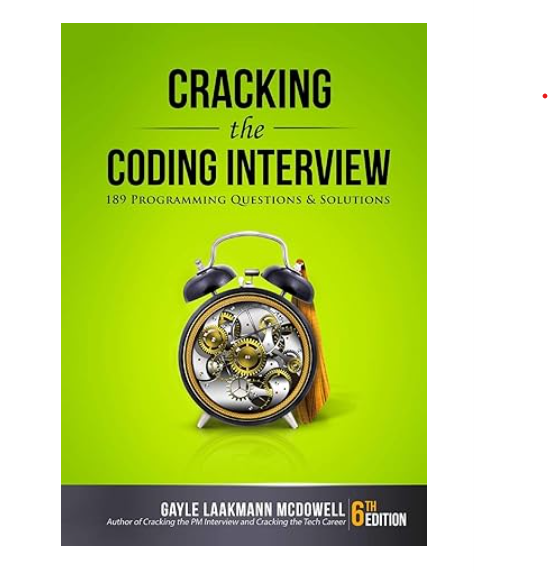
Javascript Array slice in C++ With Example Code
JavaScript’s `Array.slice()` method is a powerful tool for manipulating arrays. However, if you’re working in C++, you might be wondering how to achieve the same functionality. Fortunately, C++ provides a similar method called `std::vector::erase()`.
To use `std::vector::erase()`, you’ll need to include the `
#include
#include
int main() {
std::vector
// Erase the first two elements
myVector.erase(myVector.begin(), myVector.begin() + 2);
// Print the remaining elements
for (int i : myVector) {
std::cout << i << " ";
}
return 0;
}
In this example, we create a vector called `myVector` with the values 1 through 5. We then use `myVector.erase()` to remove the first two elements (1 and 2). Finally, we print the remaining elements (3, 4, and 5) using a for loop.
Note that `std::vector::erase()` takes two arguments: the first is an iterator pointing to the first element to be erased, and the second is an iterator pointing to one past the last element to be erased. In our example, we use `myVector.begin()` to get an iterator pointing to the first element, and we add 2 to it to get an iterator pointing to the third element (which is one past the last element we want to erase).
With `std::vector::erase()`, you can achieve the same functionality as `Array.slice()` in JavaScript. Happy coding!
Equivalent of Javascript Array slice in C++
In conclusion, the equivalent of the Javascript Array slice function in C++ is the std::vector's erase and insert functions. These functions allow us to extract a portion of the vector and create a new vector with the extracted elements. The erase function removes the elements from the original vector, while the insert function adds the extracted elements to the new vector. By using these functions, we can achieve the same functionality as the Javascript Array slice function in C++. It's important to note that the syntax and usage of these functions may differ from the Javascript Array slice function, but the end result is the same. Overall, the std::vector's erase and insert functions are a powerful tool for manipulating vectors in C++.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |