The JavaScript Array slice function is used to extract a portion of an array and return a new array with the extracted elements. It takes two arguments: the starting index and the ending index (optional). The starting index is the position of the first element to be included in the new array, while the ending index is the position of the first element to be excluded. If the ending index is not specified, all elements from the starting index to the end of the array will be included. The original array is not modified by the slice function. Keep reading below to learn how to Javascript Array slice in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
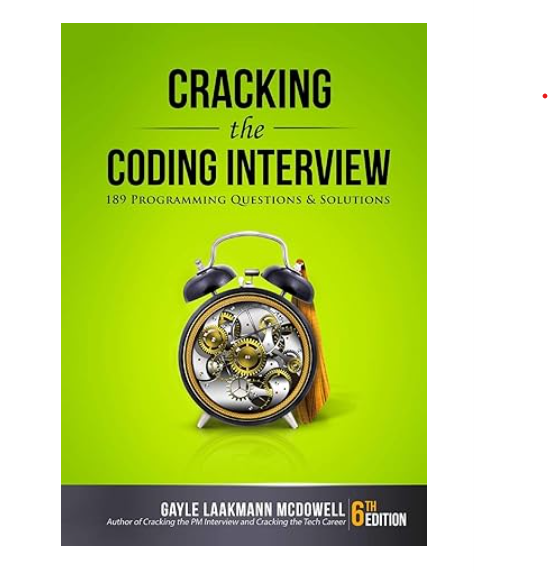
Javascript Array slice in Go With Example Code
JavaScript’s `Array.slice()` method is a powerful tool for manipulating arrays. But what if you’re working in Go and need to perform the same operation? Fortunately, Go provides a similar function that can be used to slice arrays.
The `slice` function in Go works similarly to `Array.slice()` in JavaScript. It takes an array and two indices as arguments, and returns a new slice that includes all elements between those indices.
Here’s an example of how to use `slice` in Go:
arr := [5]int{1, 2, 3, 4, 5}
slice := arr[1:4]
fmt.Println(slice) // Output: [2 3 4]
In this example, we create an array `arr` with five elements. We then use the `slice` function to create a new slice that includes elements 1 through 3 of the array (remember that Go uses zero-based indexing). Finally, we print the contents of the slice to the console.
One important thing to note is that the `slice` function in Go does not modify the original array. Instead, it creates a new slice that references a subset of the original array’s elements. This means that any changes made to the slice will not affect the original array.
Overall, the `slice` function in Go provides a powerful way to manipulate arrays and extract subsets of their elements. Whether you’re working with large datasets or just need to extract a few elements from an array, `slice` is a useful tool to have in your Go programming toolbox.
Equivalent of Javascript Array slice in Go
In conclusion, the equivalent of the Javascript Array slice function in Go is the built-in function `slice`. This function allows developers to extract a portion of an existing slice and create a new slice with the extracted elements. The `slice` function takes in three arguments: the original slice, the starting index, and the ending index (exclusive). Using the `slice` function in Go can greatly simplify the process of manipulating slices and extracting specific elements. It provides a convenient and efficient way to work with slices, making it a valuable tool for developers working with Go. Overall, the `slice` function is a powerful feature of Go that can help developers write cleaner and more efficient code. By understanding how to use this function, developers can take full advantage of the capabilities of Go and create high-quality applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |