The JavaScript Array slice function is used to extract a portion of an array and return a new array with the extracted elements. It takes two arguments: the starting index and the ending index (optional). The starting index is the position of the first element to be included in the new array, while the ending index is the position of the first element to be excluded. If the ending index is not specified, all elements from the starting index to the end of the array will be included. The original array is not modified by the slice function. Keep reading below to learn how to Javascript Array slice in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
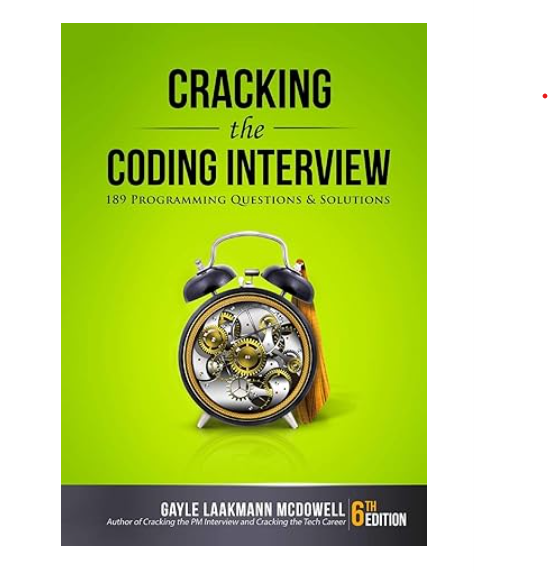
Javascript Array slice in Rust With Example Code
JavaScript developers who are transitioning to Rust may be wondering how to perform certain tasks in Rust that they are used to doing in JavaScript. One such task is slicing arrays. In JavaScript, developers can use the `slice()` method to extract a portion of an array. Rust has a similar method called `slice()` that can be used to achieve the same result.
To use the `slice()` method in Rust, you first need to create a slice from the original array. A slice is a reference to a portion of an array. You can create a slice by specifying the starting and ending indices of the portion you want to extract. Here’s an example:
let arr = [1, 2, 3, 4, 5];
let slice = &arr[1..3];
In this example, we create an array `arr` with five elements. We then create a slice `slice` that contains elements 2 and 3 of the original array. The `&` symbol before `arr[1..3]` indicates that we are creating a reference to the slice rather than copying its contents.
Once you have created a slice, you can perform operations on it just like you would with an array. For example, you can iterate over the slice using a `for` loop:
for num in slice {
println!("{}", num);
}
This will output:
2
3
You can also modify the elements in the slice:
slice[0] = 10;
This will change the first element of the slice (which corresponds to the second element of the original array) to 10.
In summary, the `slice()` method in Rust can be used to extract a portion of an array, just like the `slice()` method in JavaScript. To use it, you need to create a slice by specifying the starting and ending indices of the portion you want to extract. Once you have created a slice, you can perform operations on it just like you would with an array.
Equivalent of Javascript Array slice in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate arrays with its built-in slice functionality. The equivalent of the Javascript Array slice function in Rust allows developers to easily extract a portion of an array and perform operations on it without modifying the original array. This feature is particularly useful in scenarios where large amounts of data need to be processed or when working with complex data structures. With Rust’s slice functionality, developers can write more concise and efficient code, resulting in faster and more reliable applications. Overall, Rust’s slice functionality is a valuable tool for any developer looking to work with arrays in a high-performance language.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |