The JavaScript Array some() function is used to check if at least one element in an array satisfies a given condition. It takes a callback function as an argument that is executed for each element in the array until it finds an element that satisfies the condition. If such an element is found, the function returns true, otherwise, it returns false. The some() function is useful when you need to check if an array contains at least one element that meets a certain criteria without having to loop through the entire array. Keep reading below to learn how to Javascript Array some in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
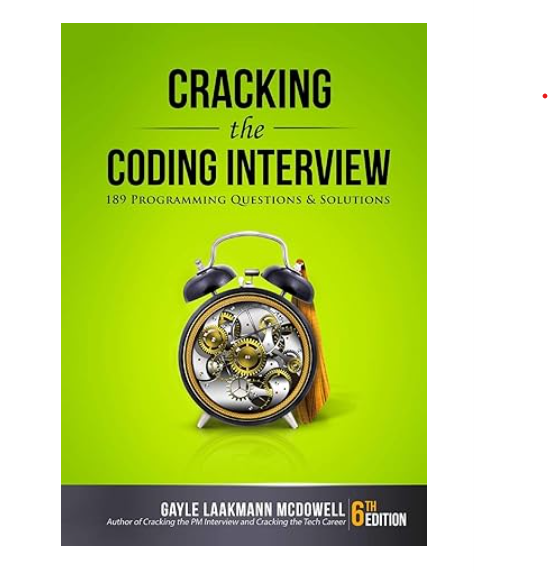
Javascript Array some in Go With Example Code
JavaScript arrays are a fundamental data structure in web development. They allow developers to store and manipulate collections of data in a simple and efficient way. In Go, arrays are also available, but they have some differences compared to JavaScript arrays. In this blog post, we will explore how to use arrays in Go and how to perform some common operations on them.
Declaring an array in Go is similar to declaring a variable. You specify the type of the array and its size. For example, to declare an array of integers with a size of 5, you would use the following code:
var myArray [5]int
This creates an array called “myArray” that can hold 5 integers. You can access individual elements of the array using their index, which starts at 0. For example, to set the first element of the array to 10, you would use the following code:
myArray[0] = 10
To initialize an array with values, you can use an array literal. An array literal is a comma-separated list of values enclosed in curly braces. For example, to create an array of strings with three elements, you would use the following code:
myArray := [3]string{"foo", "bar", "baz"}
You can also use the ellipsis notation to create an array with a variable number of elements. For example, to create an array of integers with three elements, you would use the following code:
myArray := [...]int{1, 2, 3}
To get the length of an array in Go, you can use the built-in “len” function. For example, to get the length of the “myArray” array, you would use the following code:
length := len(myArray)
To iterate over an array in Go, you can use a “for” loop. For example, to print all the elements of the “myArray” array, you would use the following code:
for i := 0; i < len(myArray); i++ {
fmt.Println(myArray[i])
}
In conclusion, arrays in Go are similar to arrays in JavaScript, but they have some differences in syntax and behavior. By understanding how to declare, initialize, and manipulate arrays in Go, you can write more efficient and effective code.
Equivalent of Javascript Array some in Go
In conclusion, the equivalent of the Javascript Array some function in Go is the `any` function from the `reflect` package. While the syntax and usage may differ slightly, both functions serve the same purpose of checking if at least one element in an array satisfies a given condition. The `any` function in Go uses reflection to dynamically determine the type of the array and perform the check, making it a versatile and powerful tool for working with arrays in Go. Whether you're a seasoned Javascript developer looking to transition to Go or a beginner just starting out, understanding the similarities and differences between these two functions can help you write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |