The JavaScript Array some() function is used to check if at least one element in an array satisfies a given condition. It takes a callback function as an argument that is executed for each element in the array until it finds an element that satisfies the condition. If such an element is found, the function returns true, otherwise, it returns false. The some() function is useful when you need to check if an array contains at least one element that meets a certain criteria without having to loop through the entire array. Keep reading below to learn how to Javascript Array some in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
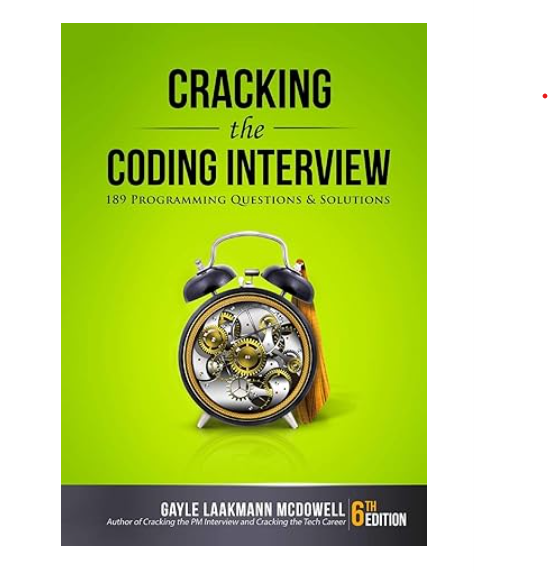
Javascript Array some in Java With Example Code
JavaScript arrays are a powerful tool for storing and manipulating data in web development. However, did you know that you can also use arrays in Java? In this blog post, we will explore how to use JavaScript arrays in Java.
First, let’s take a look at how to declare an array in Java. To declare an array, you need to specify the data type of the array and the number of elements it will contain. Here’s an example:
int[] myArray = new int[5];
This creates an integer array called “myArray” with five elements.
To add elements to the array, you can use the following syntax:
myArray[0] = 1;
This sets the first element of the array to 1. You can continue to add elements to the array in this way.
To access elements in the array, you can use the following syntax:
int element = myArray[0];
This sets the variable “element” to the value of the first element in the array.
You can also use loops to iterate over the elements in the array. Here’s an example of how to use a for loop to iterate over the elements in the array:
for (int i = 0; i < myArray.length; i++) {
System.out.println(myArray[i]);
}
This will print out each element in the array.
In conclusion, using JavaScript arrays in Java can be a useful tool for storing and manipulating data. By declaring an array, adding elements, accessing elements, and iterating over the elements, you can use JavaScript arrays in Java to make your code more efficient and effective.
Equivalent of Javascript Array some in Java
In conclusion, the equivalent of the JavaScript Array.some() function in Java is the Stream.anyMatch() method. Both functions serve the same purpose of checking if at least one element in an array or stream meets a certain condition. However, the syntax and implementation of these functions differ between the two languages. It is important for developers to understand the nuances of each language and its corresponding functions in order to write efficient and effective code. By utilizing the Stream.anyMatch() method in Java, developers can easily check for the presence of a specific element in a stream and streamline their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |