The JavaScript Array some() function is used to check if at least one element in an array satisfies a given condition. It takes a callback function as an argument that is executed for each element in the array until it finds an element that satisfies the condition. If such an element is found, the function returns true, otherwise, it returns false. The some() function is useful when you need to check if an array contains at least one element that meets a certain criteria without having to loop through the entire array. Keep reading below to learn how to Javascript Array some in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
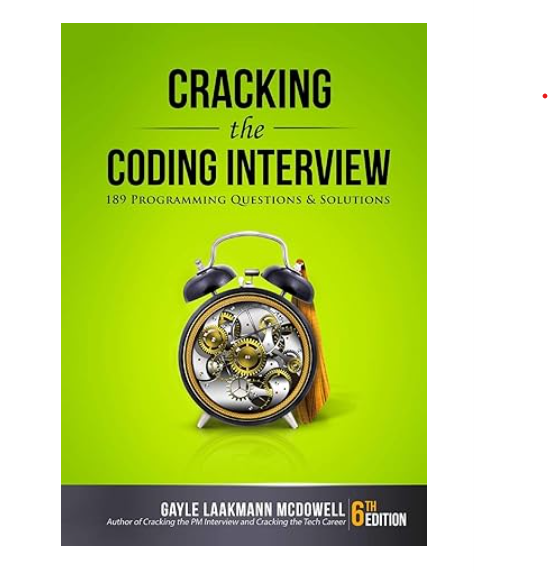
Javascript Array some in Rust With Example Code
JavaScript developers who are interested in Rust may be wondering how to use Rust’s array functionality. Rust’s arrays are fixed-size and can hold elements of the same type. Here’s how to use Rust’s array functionality to create and manipulate arrays.
To create an array in Rust, you can use the following syntax:
let my_array = [1, 2, 3, 4, 5];
This creates an array called `my_array` with five elements. You can access individual elements of the array using indexing, like this:
let first_element = my_array[0];
This sets the variable `first_element` to the first element of `my_array`.
You can also modify elements of the array using indexing:
my_array[0] = 6;
This sets the first element of `my_array` to 6.
To loop through the elements of an array in Rust, you can use a `for` loop:
for element in my_array.iter() {
println!("{}", element);
}
This will print each element of `my_array` on a new line.
You can also use Rust’s built-in array methods to manipulate arrays. For example, you can use the `len` method to get the length of an array:
let array_length = my_array.len();
This sets the variable `array_length` to the length of `my_array`.
Overall, Rust’s array functionality is similar to JavaScript’s array functionality, but with some differences in syntax and behavior. With these basics, JavaScript developers can start exploring Rust’s array capabilities.
Equivalent of Javascript Array some in Rust
In conclusion, the Rust programming language provides a powerful and efficient alternative to Javascript when it comes to working with arrays. The equivalent Rust function to Javascript’s Array.some() method, the iter.any() method, allows developers to easily check if at least one element in an array satisfies a given condition. With Rust’s focus on performance and memory safety, this function can be used to efficiently process large arrays without sacrificing speed or stability. Overall, Rust’s array manipulation capabilities make it a valuable tool for developers looking to optimize their code and improve their programming skills.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |