The JavaScript Array some() function is used to check if at least one element in an array satisfies a given condition. It takes a callback function as an argument that is executed for each element in the array until it finds an element that satisfies the condition. If such an element is found, the function returns true, otherwise, it returns false. The some() function is useful when you need to check if an array contains at least one element that meets a certain criteria without having to loop through the entire array. Keep reading below to learn how to Javascript Array some in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
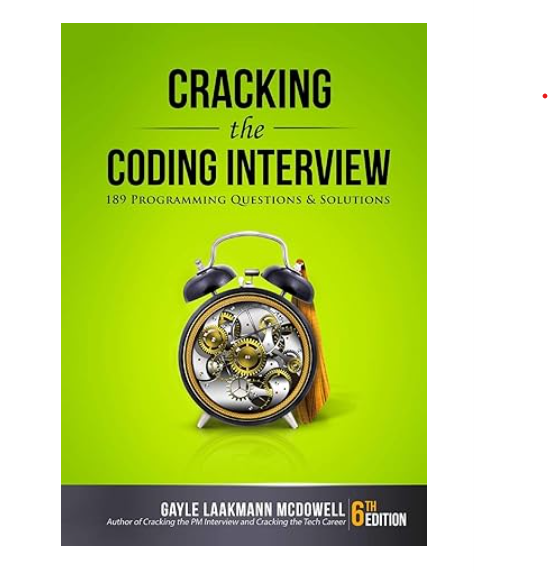
Javascript Array some in TypeScript With Example Code
JavaScript arrays are a powerful tool for storing and manipulating data. TypeScript, a superset of JavaScript, adds static typing and other features to make working with arrays even easier. In this blog post, we’ll explore how to use arrays in TypeScript.
To declare an array in TypeScript, you can use the following syntax:
let myArray: Array<string> = ['apple', 'banana', 'orange'];
This declares an array of strings and initializes it with three values. You can also declare an array using the shorthand syntax:
let myArray: string[] = ['apple', 'banana', 'orange'];
Both of these syntaxes are equivalent and can be used interchangeably.
To access an element in an array, you can use the index of the element. The index of the first element in an array is 0. For example:
let myArray: string[] = ['apple', 'banana', 'orange'];
console.log(myArray[0]); // Output: 'apple'
This code declares an array of strings and logs the first element to the console.
You can also use array methods to manipulate arrays in TypeScript. For example, you can use the push method to add an element to the end of an array:
let myArray: string[] = ['apple', 'banana', 'orange'];
myArray.push('pear');
console.log(myArray); // Output: ['apple', 'banana', 'orange', 'pear']
This code declares an array of strings, adds a new element to the end of the array using the push method, and logs the updated array to the console.
In conclusion, TypeScript arrays are a powerful tool for storing and manipulating data. By using static typing and array methods, you can write more robust and maintainable code.
Equivalent of Javascript Array some in TypeScript
In conclusion, the equivalent JavaScript Array some function in TypeScript provides a powerful tool for developers to efficiently search through arrays and determine if at least one element meets a certain condition. By using the some function, developers can write cleaner and more concise code, while also improving the performance of their applications. TypeScript’s type checking also adds an extra layer of safety and reliability to the code, ensuring that any potential errors are caught early on in the development process. Overall, the some function in TypeScript is a valuable addition to any developer’s toolkit, and can greatly enhance the functionality and efficiency of their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |