The JavaScript Array splice function is used to add or remove elements from an array. It takes three parameters: the starting index, the number of elements to remove, and any elements to add. If only the starting index is provided, all elements from that index to the end of the array are removed. If the number of elements to remove is not specified, all elements from the starting index to the end of the array are removed. If elements are added, they are inserted at the starting index and any existing elements are shifted to make room. The splice function modifies the original array and returns an array of the removed elements. Keep reading below to learn how to Javascript Array splice in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
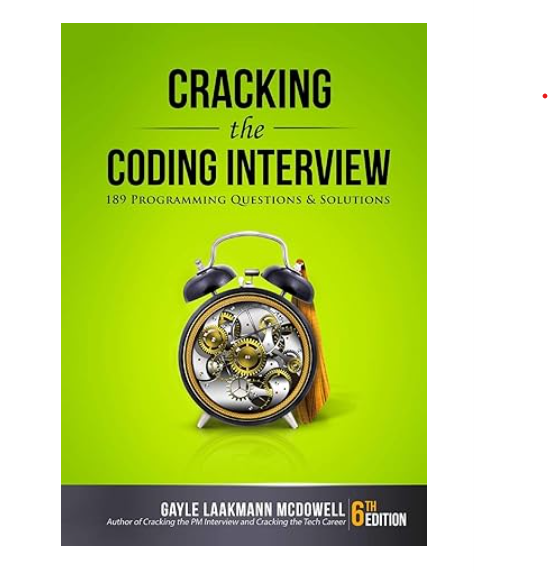
Javascript Array splice in Bash With Example Code
JavaScript’s `Array.splice()` method is a powerful tool for manipulating arrays. But did you know that you can use it in Bash as well? In this post, we’ll explore how to use `Array.splice()` in Bash to add, remove, and replace elements in an array.
To get started, let’s create an array in Bash:
my_array=(apple banana cherry)
Now, let’s say we want to add an element to the array. We can use `Array.splice()` to do this:
my_array=("${my_array[@]:0:1}" "orange" "${my_array[@]:1}")
In this example, we’re using Bash’s array slicing syntax to insert the new element “orange” at index 1. The syntax `${my_array[@]:0:1}` selects the first element of the array, and `${my_array[@]:1}` selects all the elements after the first one.
Next, let’s say we want to remove an element from the array. We can use `Array.splice()` for this as well:
my_array=("${my_array[@]:0:2}" "${my_array[@]:3}")
In this example, we’re using array slicing to remove the element at index 2. The syntax `${my_array[@]:0:2}` selects the first two elements of the array, and `${my_array[@]:3}` selects all the elements after the third one.
Finally, let’s say we want to replace an element in the array. We can use `Array.splice()` to do this as well:
my_array=("${my_array[@]:0:1}" "grape" "${my_array[@]:2}")
In this example, we’re using array slicing to replace the element at index 1 with the new element “grape”. The syntax `${my_array[@]:0:1}` selects the first element of the array, and `${my_array[@]:2}` selects all the elements after the second one.
And that’s it! With `Array.splice()`, you can easily add, remove, and replace elements in Bash arrays.
Equivalent of Javascript Array splice in Bash
In conclusion, the Bash equivalent of the Javascript Array splice function is a powerful tool for manipulating arrays in Bash scripts. By using the array slicing syntax and the `unset` command, we can easily add, remove, and replace elements in an array. This functionality is particularly useful when working with large datasets or when performing complex data manipulations. With the Bash splice function, we can streamline our scripts and make them more efficient and effective. Whether you’re a seasoned Bash programmer or just getting started, the splice function is a valuable addition to your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |