The JavaScript Array splice function is used to add or remove elements from an array. It takes three parameters: the starting index, the number of elements to remove, and any elements to add. If only the starting index is provided, all elements from that index to the end of the array are removed. If the number of elements to remove is not specified, all elements from the starting index to the end of the array are removed. If elements are added, they are inserted at the starting index and any existing elements are shifted to make room. The splice function modifies the original array and returns an array of the removed elements. Keep reading below to learn how to Javascript Array splice in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
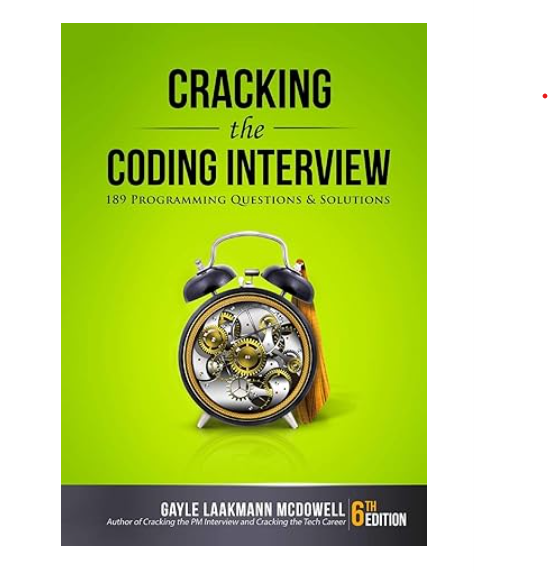
Javascript Array splice in Rust With Example Code
JavaScript’s `Array.prototype.splice()` method is a powerful tool for manipulating arrays. It allows you to add, remove, and replace elements in an array, all in one fell swoop. But what if you’re working in Rust? Fear not! Rust has its own `Vec` type that can be used in much the same way as JavaScript’s arrays, and it also has a `splice()` method.
The `Vec::splice()` method takes three arguments: the starting index of the splice, the number of elements to remove, and an iterable of elements to insert. Here’s an example:
let mut v = vec![1, 2, 3, 4, 5];
let removed = v.splice(1..4, vec![6, 7, 8]);
assert_eq!(v, vec![1, 6, 7, 8, 5]);
assert_eq!(removed, vec![2, 3, 4]);
In this example, we start by creating a `Vec` with the values 1 through 5. We then call `splice()` on the vector, passing in a range from index 1 to index 4 (which includes elements 2, 3, and 4), and a new `Vec` with the values 6, 7, and 8. The `splice()` method removes the elements at the specified range and inserts the new elements in their place. The method returns the removed elements as a new `Vec`.
Note that the `splice()` method modifies the original `Vec` in place. If you want to create a new `Vec` without modifying the original, you can use the `Vec::split_off()` method to split the original `Vec` at the splice point and return the removed elements as a new `Vec`. Here’s an example:
let mut v = vec![1, 2, 3, 4, 5];
let removed = v.split_off(1);
let mut new_v = vec![6, 7, 8];
new_v.append(&mut v);
assert_eq!(new_v, vec![6, 2, 3, 4, 5, 7, 8]);
assert_eq!(removed, vec![1]);
In this example, we start by creating a `Vec` with the values 1 through 5. We then call `split_off()` on the vector, passing in the index 1. This method splits the `Vec` at the specified index and returns the removed elements as a new `Vec`. We then create a new `Vec` with the values 6, 7, and 8, and append the original `Vec` to it using the `append()` method. The result is a new `Vec` with the elements in the desired order, and the removed element (1) in a separate `Vec`.
Equivalent of Javascript Array splice in Rust
In conclusion, the Rust programming language provides a powerful and efficient equivalent to the JavaScript Array splice function. The Vec type in Rust allows for dynamic resizing and manipulation of arrays, and the splice-like functions available in Rust’s standard library provide a range of options for modifying arrays in place. Whether you need to add or remove elements from an array, or simply rearrange its contents, Rust’s array manipulation capabilities make it a great choice for high-performance applications. So if you’re looking for a language that can handle complex array operations with ease, Rust is definitely worth considering.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |