The JavaScript Array unshift() function is used to add one or more elements to the beginning of an array. It modifies the original array and returns the new length of the array. The elements are added in the order they appear in the function arguments. This function is useful when you want to add new elements to the beginning of an array without changing the order of the existing elements. Keep reading below to learn how to Javascript Array unshift in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
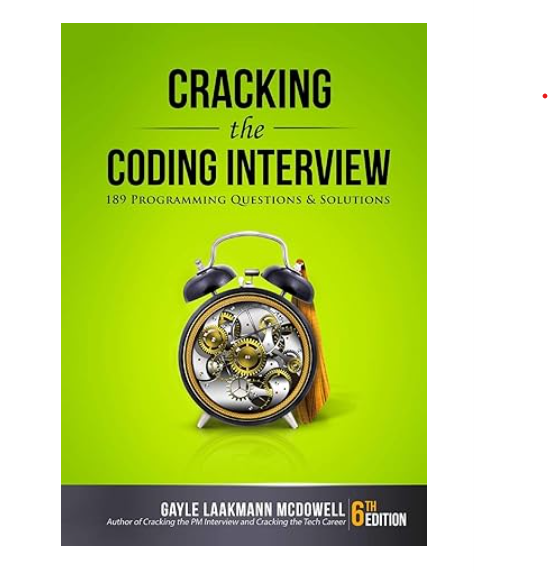
Javascript Array unshift in C++ With Example Code
JavaScript’s array method `unshift()` adds one or more elements to the beginning of an array and returns the new length of the array. C++ does not have a built-in method for this, but it can be easily implemented using pointers and dynamic memory allocation.
To implement `unshift()` in C++, we first need to create a new array with the desired elements added to the beginning. We can do this by dynamically allocating memory for a new array that is one element larger than the original array, and then copying the original array’s elements into the new array starting at index 1. Finally, we can add the new element(s) to the beginning of the new array at index 0.
Here’s an example implementation of `unshift()` in C++:
void unshift(int* &arr, int &size, int element) {
// Allocate memory for new array
int* newArr = new int[size + 1];
// Copy original array's elements into new array starting at index 1
for (int i = 0; i < size; i++) {
newArr[i + 1] = arr[i];
}
// Add new element to beginning of new array
newArr[0] = element;
// Update original array and size
delete[] arr;
arr = newArr;
size++;
}
In this implementation, `arr` is a pointer to the original array, `size` is the size of the original array, and `element` is the new element(s) to add to the beginning of the array. The function uses a reference to the pointer `arr` and the integer `size` so that the original array and size can be updated after the new array is created.
To use this function, simply call it with the original array, size, and new element(s) as arguments:
int main() {
int* arr = new int[3] {1, 2, 3};
int size = 3;
unshift(arr, size, 0);
// Output: [0, 1, 2, 3]
for (int i = 0; i < size; i++) {
std::cout << arr[i] << " ";
}
delete[] arr;
return 0;
}
In this example, the original array `[1, 2, 3]` is passed to `unshift()` along with the new element `0`. After the function is called, the original array is updated to `[0, 1, 2, 3]` and the new size is `4`. The updated array is then outputted to the console.
Equivalent of Javascript Array unshift in C++
In conclusion, the equivalent function of Javascript's Array unshift() in C++ is the std::deque::push_front() function. Both functions add an element to the beginning of an array-like data structure. However, it is important to note that while Javascript arrays are dynamic and can grow or shrink in size, C++ arrays have a fixed size. Therefore, if you need to add elements dynamically to a C++ array, you should use a container like std::deque instead. Overall, understanding the equivalent functions in different programming languages can help you become a more versatile and efficient programmer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |