The JavaScript String charCodeAt() function returns the Unicode value of the character at the specified index in a string. The index is zero-based, meaning the first character in the string has an index of 0, the second character has an index of 1, and so on. The returned value is an integer between 0 and 65535, representing the Unicode value of the character. If the specified index is out of range, the function returns NaN (Not a Number). This function is useful for working with non-ASCII characters and for performing string manipulation tasks that require knowledge of the Unicode values of characters. Keep reading below to learn how to Javascript String charCodeAt in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
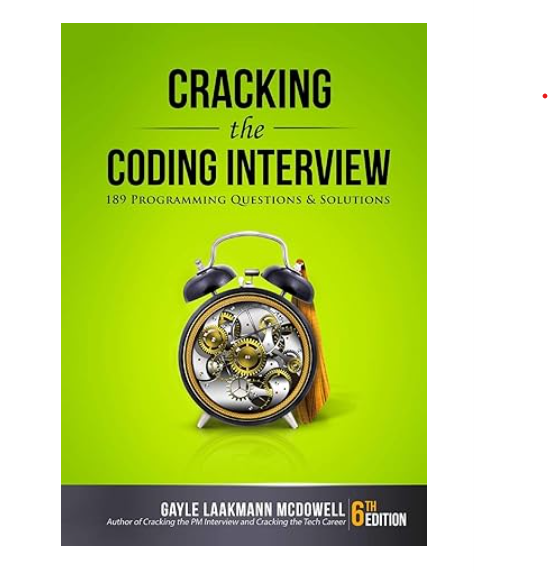
Javascript String charCodeAt in C# With Example Code
JavaScript’s `charCodeAt()` method is used to return the Unicode value of the character at a specified index in a string. In C#, we can achieve the same functionality using the `Convert.ToUInt16()` method.
Here’s an example code snippet that demonstrates how to use `Convert.ToUInt16()` to get the Unicode value of a character at a specified index in a string:
string str = "Hello, World!";
int index = 1;
ushort unicodeValue = Convert.ToUInt16(str[index]);
Console.WriteLine("The Unicode value of the character at index {0} is {1}", index, unicodeValue);
In the above code, we first define a string `str` and an integer `index` to represent the index of the character whose Unicode value we want to find. We then use the `Convert.ToUInt16()` method to convert the character at the specified index to its corresponding Unicode value, which is stored in the `unicodeValue` variable. Finally, we print out the index and Unicode value of the character using the `Console.WriteLine()` method.
By using the `Convert.ToUInt16()` method, we can easily get the Unicode value of any character in a string in C#.
Equivalent of Javascript String charCodeAt in C#
In conclusion, the equivalent function of Javascript’s String charCodeAt in C# is the Char.ConvertToUtf32 method. This method allows developers to retrieve the UTF-32 code point of a specified character in a string. While the syntax and usage may differ slightly between the two languages, the functionality remains the same. As a developer, it is important to understand the similarities and differences between programming languages and their functions in order to write efficient and effective code. By utilizing the Char.ConvertToUtf32 method in C#, developers can easily retrieve the code point of a character in a string and use it in their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |