The JavaScript String concat() function is used to concatenate two or more strings and return a new string. It takes one or more string arguments and joins them together in the order they are provided. The original strings are not modified, and the resulting concatenated string is returned. The concat() function can be called on any string object or used as a standalone function. It is a useful tool for combining strings in JavaScript programming. Keep reading below to learn how to Javascript String concat in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
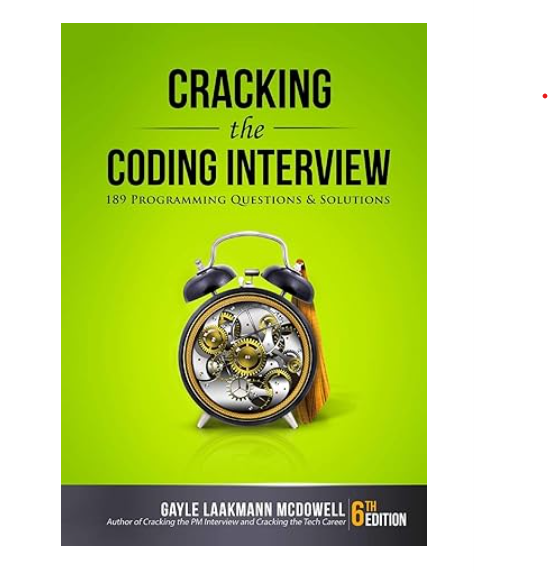
Javascript String concat in Rust With Example Code
If you’re familiar with JavaScript, you may be used to using the concat()
method to concatenate strings. In Rust, you can achieve the same result using the format!
macro.
The format!
macro allows you to create a formatted string by combining multiple values. Here’s an example:
let name = "John";
let age = 30;
let message = format!("My name is {} and I am {} years old.", name, age);
println!("{}", message);
In this example, we’re creating a string that includes the values of the name
and age
variables. The format!
macro replaces the {}
placeholders with the values of the variables.
You can also use the to_string()
method to convert a value to a string and concatenate it with another string. Here’s an example:
let name = "John";
let message = "My name is ".to_string() + name;
println!("{}", message);
In this example, we’re using the to_string()
method to convert the "My name is "
string to a String
object. We’re then using the +
operator to concatenate the name
variable with the String
object.
Equivalent of Javascript String concat in Rust
In conclusion, Rust provides a powerful and efficient way to concatenate strings using the `String::push_str` function. This function allows developers to append one string to another without creating a new string object, which can save memory and improve performance. Additionally, Rust’s ownership and borrowing system ensures that the strings are handled safely and efficiently. While the syntax may be different from other programming languages like JavaScript, the `String::push_str` function in Rust is a reliable and effective way to concatenate strings. As Rust continues to gain popularity among developers, it’s important to understand the language’s unique features and capabilities, including its string manipulation functions.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |