The JavaScript String indexOf function is used to find the index of a specified substring within a string. It takes one or two arguments, the first being the substring to search for and the second being an optional starting index. If the substring is found, the function returns the index of the first occurrence of the substring within the string. If the substring is not found, the function returns -1. The function is case-sensitive, meaning that it will only match substrings that have the same case as the search string. Keep reading below to learn how to Javascript String indexOf in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
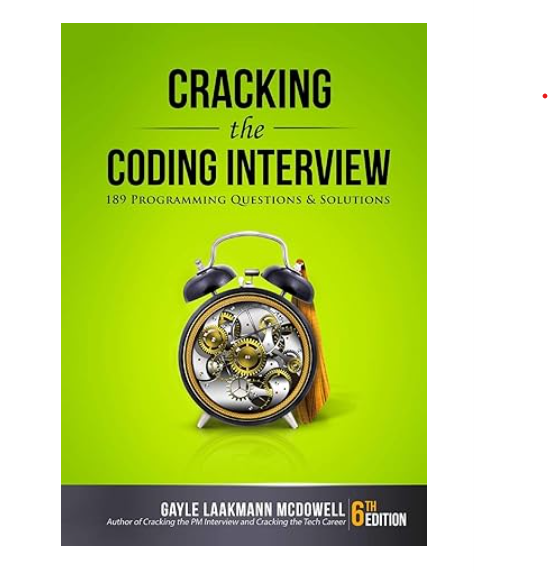
Javascript String indexOf in C++ With Example Code
JavaScript’s `indexOf()` method is a useful tool for finding the index of a specific character or substring within a string. However, if you’re working with C++, you may be wondering how to achieve the same functionality. Fortunately, C++ provides a similar method called `find()` that can be used to accomplish this task.
To use `find()`, you’ll need to include the `
Here’s an example of how to use `find()` in C++:
#include
#include
int main() {
std::string str = "Hello, world!";
std::string substr = "world";
size_t index = str.find(substr);
if (index != std::string::npos) {
std::cout << "Substring found at index " << index << std::endl;
} else {
std::cout << "Substring not found" << std::endl;
}
return 0;
}
In this example, we create a string `str` and a substring `substr` that we want to find within `str`. We then call `find()` on `str` and pass in `substr` as an argument. If `substr` is found within `str`, `find()` will return the index of the first occurrence of `substr`. We then check if the index is not equal to `std::string::npos`, which indicates that `substr` was not found. If `substr` was found, we print out the index at which it was found.
Using `find()` in C++ is a simple and effective way to achieve the same functionality as JavaScript's `indexOf()` method.
Equivalent of Javascript String indexOf in C++
In conclusion, the equivalent function of Javascript's String indexOf in C++ is the find() function. Both functions serve the same purpose of searching for a specific substring within a given string. However, there are some differences in their syntax and usage. While the String indexOf function returns -1 if the substring is not found, the find() function returns the string::npos constant. Additionally, the find() function can also be used with other data types such as vectors and arrays. Overall, the find() function is a powerful tool in C++ for searching and manipulating strings, and it is a great alternative to the String indexOf function in Javascript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |