The JavaScript String indexOf function is used to find the index of a specified substring within a string. It takes one or two arguments, the first being the substring to search for and the second being an optional starting index. If the substring is found, the function returns the index of the first occurrence of the substring within the string. If the substring is not found, the function returns -1. The function is case-sensitive, meaning that it will only match substrings that have the same case as the search string. The indexOf function is commonly used in JavaScript to search for specific characters or words within a string and to manipulate strings based on their contents. Keep reading below to learn how to Javascript String indexOf in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
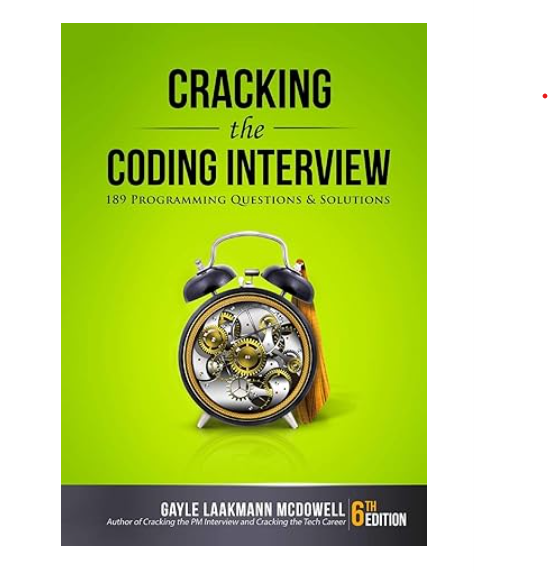
Javascript String indexOf in Kotlin With Example Code
JavaScript’s `indexOf()` method is a useful tool for finding the index of a specific character or substring within a string. In Kotlin, we can achieve the same functionality using the `indexOf()` method provided by the `String` class.
To use the `indexOf()` method in Kotlin, we simply call it on a string object and pass in the character or substring we want to find the index of. The method returns the index of the first occurrence of the character or substring, or -1 if it is not found.
Here’s an example of using the `indexOf()` method in Kotlin:
val str = "Hello, world!"
val index = str.indexOf("o")
println(index) // Output: 4
In this example, we create a string `str` and call the `indexOf()` method on it, passing in the character “o”. The method returns the index of the first occurrence of “o” in the string, which is 4.
We can also use the `indexOf()` method to find the index of a substring within a string. Here’s an example:
val str = "The quick brown fox jumps over the lazy dog"
val index = str.indexOf("brown")
println(index) // Output: 10
In this example, we create a string `str` and call the `indexOf()` method on it, passing in the substring “brown”. The method returns the index of the first occurrence of “brown” in the string, which is 10.
Overall, the `indexOf()` method in Kotlin provides a simple and efficient way to find the index of a character or substring within a string.
Equivalent of Javascript String indexOf in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to search for substrings within a string using the `indexOf` function. This function works similarly to the `indexOf` function in JavaScript, allowing developers to easily find the position of a substring within a larger string. With its intuitive syntax and robust functionality, Kotlin’s `indexOf` function is a valuable tool for any developer working with strings in their code. Whether you’re building a web application or a mobile app, Kotlin’s `indexOf` function can help you quickly and easily search for and manipulate strings in your code. So, if you’re looking for a reliable and efficient way to search for substrings in Kotlin, be sure to check out the `indexOf` function and see how it can help you streamline your code and improve your development workflow.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |