The JavaScript String indexOf function is used to find the index of a specified substring within a string. It takes one or two arguments, the first being the substring to search for and the second being an optional starting index. If the substring is found, the function returns the index of the first occurrence of the substring within the string. If the substring is not found, the function returns -1. The function is case-sensitive, meaning that it will only match substrings that have the same case as the search string. The indexOf function is commonly used in JavaScript to search for specific characters or words within a string and to manipulate strings based on their contents. Keep reading below to learn how to Javascript String indexOf in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
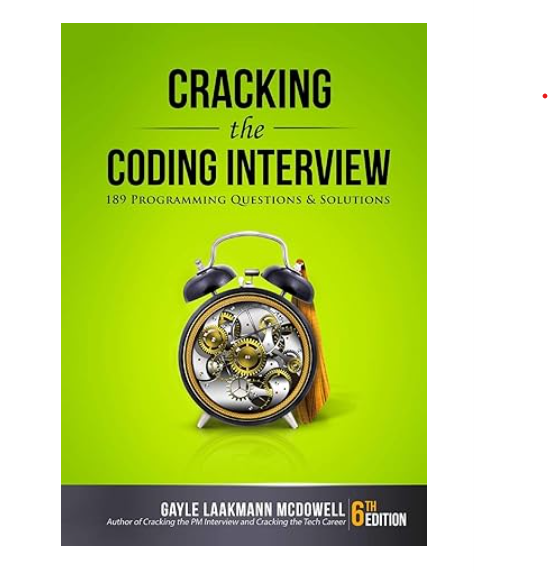
Javascript String indexOf in Python With Example Code
JavaScript and Python are two of the most popular programming languages in the world. While they have many similarities, there are also some differences between the two. One of these differences is the way they handle strings. In JavaScript, the `indexOf()` method is used to find the index of a substring within a string. In Python, there is no direct equivalent to this method, but there are several ways to achieve the same result.
One way to find the index of a substring in Python is to use the `find()` method. This method returns the index of the first occurrence of the substring within the string, or -1 if the substring is not found. Here is an example:
string = "Hello, world!"
substring = "world"
index = string.find(substring)
print(index)
This code will output `7`, which is the index of the substring “world” within the string “Hello, world!”.
Another way to find the index of a substring in Python is to use regular expressions. Regular expressions are a powerful tool for working with strings, and Python has built-in support for them through the `re` module. Here is an example:
import re
string = "Hello, world!"
substring = "world"
pattern = re.compile(substring)
match = pattern.search(string)
index = match.start()
print(index)
This code will also output `7`, which is the index of the substring “world” within the string “Hello, world!”.
In conclusion, while there is no direct equivalent to the JavaScript `indexOf()` method in Python, there are several ways to achieve the same result. The `find()` method and regular expressions are two common approaches that can be used to find the index of a substring within a string in Python.
Equivalent of Javascript String indexOf in Python
In conclusion, the Python programming language provides a similar function to the JavaScript String indexOf function. The Python equivalent is the find() method, which returns the index of the first occurrence of a substring within a string. Both functions are useful for searching for specific characters or substrings within a larger string. However, it is important to note that there are some differences between the two functions, such as the fact that the JavaScript function returns -1 if the substring is not found, while the Python function returns -1. Overall, understanding the similarities and differences between these two functions can help developers write more efficient and effective code in both languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |