The JavaScript String indexOf function is used to find the index of a specified substring within a string. It takes one or two arguments, the first being the substring to search for and the second being an optional starting index. If the substring is found, the function returns the index of the first occurrence of the substring within the string. If the substring is not found, the function returns -1. The function is case-sensitive, meaning that it will only match substrings that have the same case as the search string. The indexOf function is commonly used in JavaScript to search for specific characters or words within a string and to manipulate strings based on their contents. Keep reading below to learn how to Javascript String indexOf in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
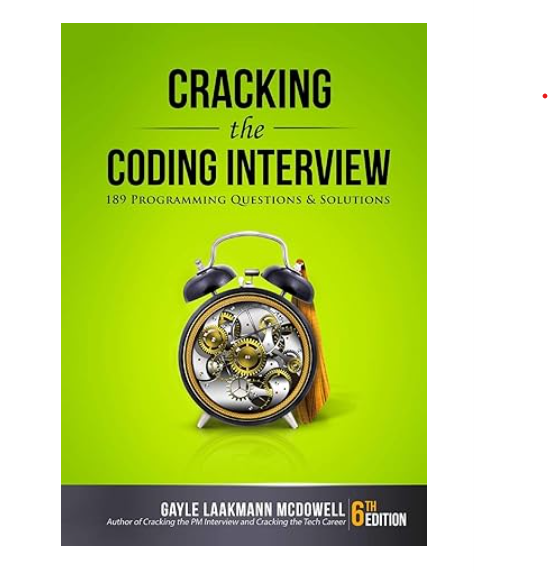
Javascript String indexOf in TypeScript With Example Code
JavaScript’s `indexOf()` method is a useful tool for finding the index of a specific character or substring within a string. In TypeScript, this method can be used in the same way as in JavaScript.
To use `indexOf()` in TypeScript, first declare a string variable and assign it a value:
let myString: string = "Hello, world!";
Then, call the `indexOf()` method on the string variable and pass in the character or substring you want to find:
let index: number = myString.indexOf("world");
This will return the index of the first occurrence of the substring “world” within the string “Hello, world!”. If the substring is not found, `indexOf()` will return -1.
It’s important to note that `indexOf()` is case-sensitive, so if you’re searching for a substring with a specific case, make sure to match the case exactly.
In addition to searching for substrings, `indexOf()` can also be used to search for individual characters within a string. For example:
let index: number = myString.indexOf("o");
This will return the index of the first occurrence of the character “o” within the string “Hello, world!”.
Overall, `indexOf()` is a powerful method for searching within strings in TypeScript, and can be used in a variety of different scenarios.
Equivalent of Javascript String indexOf in TypeScript
In conclusion, the TypeScript String indexOf function is the equivalent of the JavaScript String indexOf function, with the added benefit of type checking and improved code readability. By using TypeScript, developers can ensure that their code is more robust and less prone to errors, while still enjoying the flexibility and power of JavaScript. Whether you are a seasoned developer or just starting out, TypeScript is a valuable tool that can help you write better, more reliable code. So if you haven’t already, give TypeScript a try and see how it can improve your development workflow.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |