The lastIndexOf() function in JavaScript is a method that is used to search for a specified string within a given string and returns the index of the last occurrence of the specified string. It takes in a string as an argument and searches for the last occurrence of that string within the given string. If the specified string is found, the function returns the index of the last occurrence of the string. If the specified string is not found, the function returns -1. The lastIndexOf() function is useful when you need to find the position of the last occurrence of a specific character or substring within a string. Keep reading below to learn how to Javascript String lastIndexOf in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
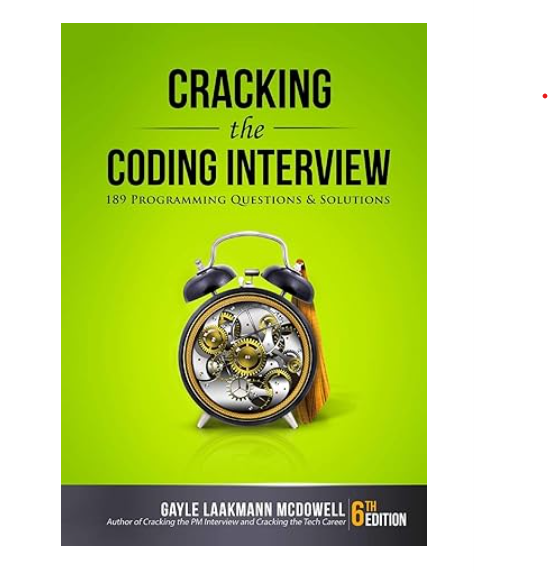
Javascript String lastIndexOf in C++ With Example Code
JavaScript’s `lastIndexOf()` method is a useful tool for finding the last occurrence of a specified string within another string. If you’re working with C++, you might be wondering if there’s an equivalent function available. Fortunately, there is! In this post, we’ll take a look at how to use the `std::string::find_last_of()` function to achieve the same result.
The `std::string::find_last_of()` function takes a string as its argument and returns the position of the last occurrence of that string within the calling string. If the string is not found, the function returns `std::string::npos`.
Here’s an example of how to use `std::string::find_last_of()`:
#include
#include
int main() {
std::string str = "Hello, world!";
std::string search = "o";
size_t pos = str.find_last_of(search);
if (pos != std::string::npos) {
std::cout << "Last occurrence of '" << search << "' found at position " << pos << std::endl;
} else {
std::cout << "String not found" << std::endl;
}
return 0;
}
In this example, we're searching for the last occurrence of the letter "o" within the string "Hello, world!". The `find_last_of()` function returns the position of the last "o", which is 8. We then output a message indicating the position of the last occurrence.
Keep in mind that `std::string::find_last_of()` searches for the last occurrence of any character in the specified string. If you want to search for a specific substring, you can use `std::string::find_last_of()` in combination with `std::string::substr()`.
Overall, `std::string::find_last_of()` is a powerful tool for finding the last occurrence of a string within another string in C++.
Equivalent of Javascript String lastIndexOf in C++
In conclusion, the equivalent C++ function for the Javascript String lastIndexOf function is the std::string::find_last_of() function. This function searches for the last occurrence of a character or substring within a given string and returns its index. It is a useful tool for manipulating strings in C++ and can be used in a variety of applications. By understanding the similarities and differences between these two functions, developers can effectively use them to achieve their desired results. Whether you are a seasoned C++ programmer or just starting out, the std::string::find_last_of() function is a valuable addition to your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |