The JavaScript String length function is a built-in method that returns the number of characters in a string. It is a property of the string object and can be accessed using dot notation. The length function counts all characters in the string, including spaces and special characters. It is useful for checking the length of user input or for manipulating strings in various ways. The length function returns an integer value representing the number of characters in the string. Keep reading below to learn how to Javascript String length in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
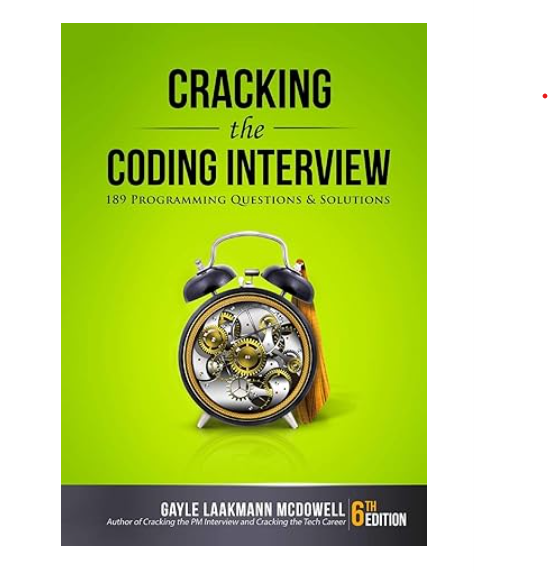
Javascript String length in C++ With Example Code
JavaScript is a popular programming language used for web development. One of the most common tasks in JavaScript is working with strings. In C++, you can also work with strings and find their length using the length()
function.
The length()
function is a built-in function in C++ that returns the length of a string. It can be used with any string variable or string literal.
Here is an example code that demonstrates how to use the length()
function:
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "Hello World!";
int len = str.length();
cout << "The length of the string is: " << len << endl;
return 0;
}
In this example, we first declare a string variable str
and assign it the value “Hello World!”. We then use the length()
function to find the length of the string and store it in an integer variable len
. Finally, we print the length of the string using the cout
statement.
Using the length()
function in C++ is a simple and effective way to find the length of a string. It can be used in a variety of applications, from simple string manipulation to more complex algorithms.
Equivalent of Javascript String length in C++
In conclusion, the equivalent C++ function for the Javascript String length function is the `strlen()` function. This function is a part of the C++ standard library and is used to determine the length of a null-terminated string. It works by counting the number of characters in the string until it reaches the null character ‘\0’. The `strlen()` function is a simple and efficient way to determine the length of a string in C++. It is important to note that the `strlen()` function only works with null-terminated strings and may not work with other types of strings. Overall, the `strlen()` function is a useful tool for C++ programmers who need to work with strings and want to determine their length quickly and easily.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |