The JavaScript String localeCompare function is used to compare two strings based on the language and cultural conventions of a specific locale. It returns a number indicating whether the first string comes before, after, or is equal to the second string in the sort order of the locale. The function takes an optional parameter that specifies the locale to use for the comparison. If no locale is specified, the function uses the default locale of the user’s environment. This function is useful for sorting and searching strings in a way that is appropriate for the user’s language and cultural preferences. Keep reading below to learn how to Javascript String localeCompare in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
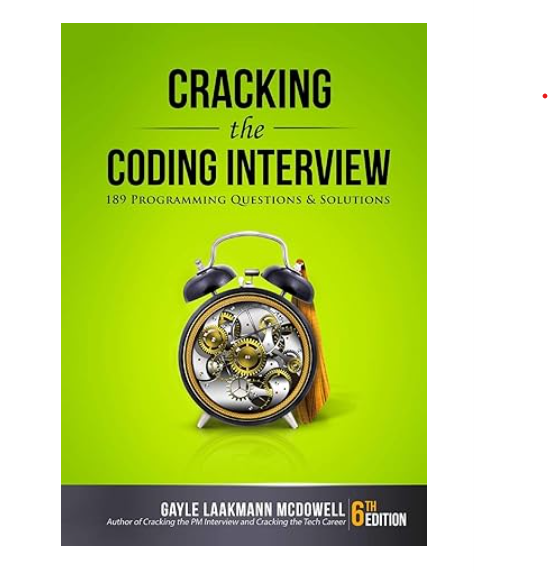
Javascript String localeCompare in C++ With Example Code
JavaScript’s `localeCompare()` method is used to compare two strings based on the language and region-specific collation order. This method is useful when sorting strings in a way that is appropriate for a particular language or region. In this blog post, we will explore how to use the `localeCompare()` method in C++.
To use the `localeCompare()` method in C++, we need to include the `
Once we have a `std::locale` object, we can use the `std::use_facet` function to get a reference to the `std::collate` facet for that locale. The `std::collate` facet provides the `compare()` method, which we can use to compare two strings based on the collation order of the locale.
Here is an example code snippet that demonstrates how to use the `localeCompare()` method in C++:
#include
#include
int main() {
std::locale loc("en_US.UTF-8");
std::string str1 = "apple";
std::string str2 = "banana";
std::collate
int result = coll.compare(str1.data(), str1.data() + str1.length(),
str2.data(), str2.data() + str2.length());
if (result < 0) {
std::cout << str1 << " comes before " << str2 << std::endl;
} else if (result > 0) {
std::cout << str2 << " comes before " << str1 << std::endl;
} else {
std::cout << str1 << " and " << str2 << " are equivalent" << std::endl;
}
return 0;
}
In this example, we create a `std::locale` object for the "en_US.UTF-8" locale, which represents the English language and the United States region. We then create two strings, "apple" and "banana", and use the `std::collate` facet to compare them based on the collation order of the locale. The `compare()` method returns a negative value if the first string comes before the second string, a positive value if the first string comes after the second string, and zero if the two strings are equivalent.
In conclusion, the `localeCompare()` method in JavaScript is a powerful tool for comparing strings based on the collation order of a specific language and region. By using the `std::locale` and `std::collate` classes in C++, we can achieve the same functionality and sort strings in a way that is appropriate for a particular locale.
Equivalent of Javascript String localeCompare in C++
In conclusion, the C++ programming language provides a powerful and efficient way to compare strings using the localeCompare function. This function allows developers to compare strings based on their cultural and linguistic context, ensuring that their applications are more accurate and reliable. By using the localeCompare function in C++, developers can easily compare strings in a way that is consistent with the user's language and cultural preferences. This can be especially useful in international applications where users may be using different languages and character sets. Overall, the localeCompare function in C++ is a valuable tool for developers who want to create high-quality, culturally-aware applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |