The JavaScript String localeCompare function is used to compare two strings based on the language and cultural conventions of a specific locale. It returns a number indicating whether the first string comes before, after, or is equal to the second string in the sort order of the locale. The function takes an optional parameter that specifies the locale to use for the comparison. If no locale is specified, the function uses the default locale of the environment. The localeCompare function is useful for sorting and searching strings in multilingual applications where the sort order may vary depending on the language and cultural conventions of the user. Keep reading below to learn how to Javascript String localeCompare in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
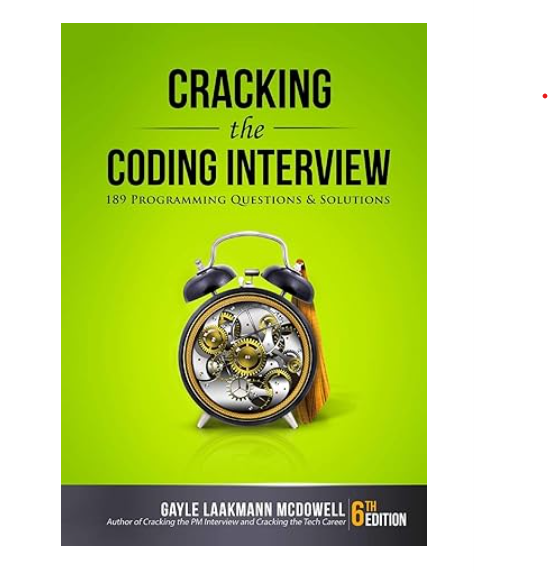
Javascript String localeCompare in Kotlin With Example Code
JavaScript’s `localeCompare()` method is a useful tool for comparing strings in a locale-sensitive way. In Kotlin, we can use the `java.util.Locale` class to achieve the same functionality.
To use `localeCompare()` in Kotlin, we first need to create a `Locale` object that represents the desired locale. We can do this by calling the `Locale` constructor with the appropriate language and country codes:
“`kotlin
val locale = Locale(“en”, “US”)
“`
Once we have our `Locale` object, we can call the `compare()` method on our two strings, passing in the `Locale` object as a parameter:
“`kotlin
val result = string1.compare(string2, locale)
“`
The `compare()` method returns an integer value that indicates the relative order of the two strings. If `string1` comes before `string2` in the specified locale, the result will be a negative number. If `string1` comes after `string2`, the result will be a positive number. If the two strings are equal in the specified locale, the result will be zero.
Here’s an example that demonstrates the use of `localeCompare()` in Kotlin:
“`kotlin
val string1 = “apple”
val string2 = “banana”
val locale = Locale(“en”, “US”)
val result = string1.compare(string2, locale)
if (result < 0) {
println("$string1 comes before $string2 in the specified locale.")
} else if (result > 0) {
println(“$string1 comes after $string2 in the specified locale.”)
} else {
println(“$string1 and $string2 are equal in the specified locale.”)
}
“`
In this example, the output would be “apple comes before banana in the specified locale.” because “apple” comes before “banana” in alphabetical order in the English language.
Equivalent of Javascript String localeCompare in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to compare strings using the `compareTo()` function. This function is similar to the `localeCompare()` function in JavaScript, allowing developers to compare strings based on their Unicode values. With the ability to specify the locale and ignore case sensitivity, the `compareTo()` function in Kotlin provides a flexible and customizable way to compare strings in a variety of scenarios. Whether you are working on a small project or a large-scale application, the `compareTo()` function in Kotlin is a valuable tool for any developer looking to compare strings with ease and accuracy.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |