The JavaScript String match() function is used to search a string for a specified pattern and returns an array of all the matches found. The pattern can be a regular expression or a string. If the pattern is a string, it searches for the exact match of the string. If the pattern is a regular expression, it searches for all the matches that match the regular expression. The match() function returns null if no match is found. The returned array contains the matched string, the index of the match, and the original string. The match() function is case-sensitive by default, but it can be made case-insensitive by using the “i” flag in the regular expression. Keep reading below to learn how to Javascript String match in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
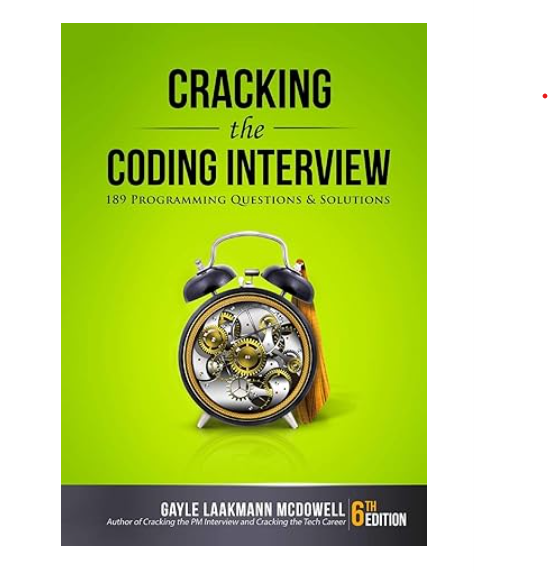
Javascript String match in C# With Example Code
Here is the HTML body for a blog post on how to use Javascript String match in C#:
If you’re familiar with Javascript, you may have used the match()
method to search for a pattern within a string. In C#, you can achieve the same functionality using the Regex.Match()
method.
The Regex.Match()
method takes two parameters: the string to search, and the regular expression pattern to match against. Here’s an example:
string input = "The quick brown fox jumps over the lazy dog.";
string pattern = "quick";
Match match = Regex.Match(input, pattern);
if (match.Success)
{
Console.WriteLine("Match found: " + match.Value);
}
In this example, we’re searching for the word “quick” within the input string. The Match()
method returns a Match
object, which contains information about the match. We can check if a match was found using the Success
property, and retrieve the matched string using the Value
property.
You can also use regular expression patterns to search for more complex patterns within a string. For example, to search for all occurrences of a word that starts with the letter “q”, you could use the following pattern:
string pattern = @"\bq\w+";
The \b
character represents a word boundary, and the \w+
pattern matches one or more word characters (letters, digits, or underscores).
Using the Regex.Match()
method in C# allows you to perform powerful string matching operations, similar to those available in Javascript. Give it a try in your next C# project!
Equivalent of Javascript String match in C#
In conclusion, the C# programming language provides a powerful and efficient equivalent to the Javascript String match function. The Regex.Match method in C# allows developers to easily search for patterns within a string and extract the desired information. With its robust set of features and options, Regex.Match is a valuable tool for any C# developer working with string manipulation. Whether you’re building a web application or a desktop application, understanding how to use Regex.Match effectively can help you write cleaner, more efficient code. So, if you’re looking to take your C# programming skills to the next level, be sure to explore the many capabilities of Regex.Match.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |