The JavaScript String match function is used to search a string for a specified pattern and returns an array of all the matches found. The pattern can be a regular expression or a string. If the pattern is a string, it searches for the exact match of the string. If the pattern is a regular expression, it searches for all the matches that fit the pattern. The match function returns null if no match is found. The match function can also be used with the global flag to search for all the matches in a string. Keep reading below to learn how to Javascript String match in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
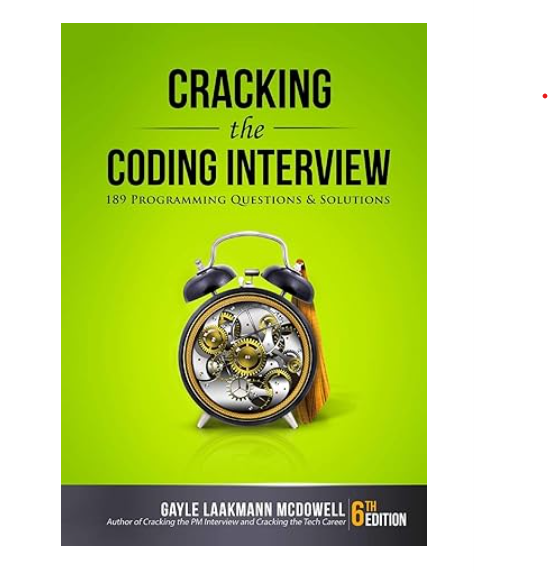
Javascript String match in Go With Example Code
JavaScript’s `String.match()` method is a powerful tool for finding matches within a string. But what if you’re working in Go? Fortunately, Go has its own built-in regular expression package that can accomplish the same task.
To use regular expressions in Go, you’ll need to import the `regexp` package. Here’s an example of how to use it to match a string:
package main
import (
"fmt"
"regexp"
)
func main() {
re := regexp.MustCompile("hello")
fmt.Println(re.MatchString("hello world"))
}
In this example, we’re using the `MatchString()` method to check if the string “hello world” contains the word “hello”. The `regexp.MustCompile()` function compiles the regular expression pattern into a regular expression object that can be used with the `MatchString()` method.
You can also use regular expression capture groups to extract specific parts of a string. Here’s an example:
package main
import (
"fmt"
"regexp"
)
func main() {
re := regexp.MustCompile(`(\d{4})-(\d{2})-(\d{2})`)
match := re.FindStringSubmatch("Today is 2022-01-01")
fmt.Println(match[1], match[2], match[3])
}
In this example, we’re using the regular expression `(\d{4})-(\d{2})-(\d{2})` to match a date in the format “YYYY-MM-DD”. The `FindStringSubmatch()` method returns an array of strings that includes the entire matched string as well as each capture group. We’re then using array indexing to extract the year, month, and day from the match.
With the `regexp` package, you can perform powerful string matching and manipulation in Go.
Equivalent of Javascript String match in Go
In conclusion, the equivalent function of Javascript’s String match in Go is the regexp package. This package provides a powerful and flexible way to match regular expressions against strings. It allows developers to define complex patterns and search for them in a given string. The regexp package also provides various methods to extract and manipulate the matched strings. With its efficient and easy-to-use syntax, the regexp package is a great tool for developers who want to perform string matching operations in Go. Overall, the regexp package is a valuable addition to the Go language, and it can help developers to write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |